#!/usr/bin/env python3 import os import sys import cv2 from pyzbar import pyzbar def main(image_folder_path, output_file_name): img_files = [f for f in os.listdir(image_folder_path) if f.endswith(('.png'))] qr_codes_found = [] for img_file in img_files: img_path = os.path.join(image_folder_path,img_file) img = cv2.imread(img_path) barcodes = pyzbar.decode(img) for barcode in barcodes: if barcode.type == 'QRCODE': qr_data = barcode.data.decode("utf-8") qr_codes_found.append((img_file, qr_data)) with open(output_file_name,'w') as f: for file_name,qr_content in qr_codes_found: f.write(f"{file_name}: {qr_content}\n") if name == "main": image_folder_path = 'home' output_file_name = 'qr_codes_found.txt' main(image_folder_path,output_file_name) 运行后报错FileNotFoundError: [Errno 2] No such file or directory: 'home'
时间: 2024-04-11 14:27:13 浏览: 178
该错误是由于找不到指定的文件夹路径而引起的。请确保您提供的图像文件夹路径是正确的,并且在运行脚本之前已经存在。
根据您的代码,您将图像文件夹路径设置为'home'。这可能是一个相对路径,如果是这样,请确保当前工作目录是包含该文件夹的文件夹。否则,您可以尝试使用绝对路径来指定图像文件夹路径。
另外,请注意,在调用`main`函数之前,您需要将代码放在一个名为`if __name__ == "__main__":`的条件语句块中。这将确保在作为脚本运行时才执行`main`函数。
以下是更新后的代码示例:
```python
#!/usr/bin/env python3
import os
import sys
import cv2
from pyzbar import pyzbar
def main(image_folder_path, output_file_name):
img_files = [f for f in os.listdir(image_folder_path) if f.endswith(('.png'))]
qr_codes_found = []
for img_file in img_files:
img_path = os.path.join(image_folder_path,img_file)
img = cv2.imread(img_path)
barcodes = pyzbar.decode(img)
for barcode in barcodes:
if barcode.type == 'QRCODE':
qr_data = barcode.data.decode("utf-8")
qr_codes_found.append((img_file, qr_data))
with open(output_file_name,'w') as f:
for file_name,qr_content in qr_codes_found:
f.write(f"{file_name}: {qr_content}\n")
if __name__ == "__main__":
image_folder_path = '/path/to/your/image/folder'
output_file_name = 'qr_codes_found.txt'
main(image_folder_path,output_file_name)
```
请将`/path/to/your/image/folder`替换为您的实际图像文件夹路径。
阅读全文
相关推荐
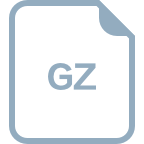











