winform跑马灯
时间: 2024-01-14 18:03:21 浏览: 30
以下是实现WinForm跑马灯效果的方法:
1. 使用Timer控件和Label控件实现跑马灯效果:
```csharp
using System;
using System.Windows.Forms;
namespace WindowsFormsApp
{
public partial class Form1 : Form
{
private Timer timer;
private Label label;
public Form1()
{
InitializeComponent();
InitializeTimer();
InitializeLabel();
}
private void InitializeTimer()
{
timer = new Timer();
timer.Interval = 100; // 设置定时器间隔时间,单位为毫秒
timer.Tick += Timer_Tick;
timer.Start();
}
private void InitializeLabel()
{
label = new Label();
label.Text = "这是一个跑马灯效果的Label";
label.Location = new System.Drawing.Point(10, 10);
label.AutoSize = true;
Controls.Add(label);
}
private void Timer_Tick(object sender, EventArgs e)
{
// 每次定时器触发时,将Label的位置向左移动一定距离
label.Left -= 5;
// 当Label完全移出窗口时,将其重新放置到窗口右侧
if (label.Right < 0)
{
label.Left = Width;
}
}
}
}
```
2. 使用PictureBox控件和Timer控件实现跑马灯效果:
```csharp
using System;
using System.Windows.Forms;
namespace WindowsFormsApp
{
public partial class Form1 : Form
{
private Timer timer;
private PictureBox pictureBox;
public Form1()
{
InitializeComponent();
InitializeTimer();
InitializePictureBox();
}
private void InitializeTimer()
{
timer = new Timer();
timer.Interval = 100; // 设置定时器间隔时间,单位为毫秒
timer.Tick += Timer_Tick;
timer.Start();
}
private void InitializePictureBox()
{
pictureBox = new PictureBox();
pictureBox.Image = Properties.Resources.marquee_image; // 设置跑马灯图片
pictureBox.SizeMode = PictureBoxSizeMode.AutoSize;
pictureBox.Location = new System.Drawing.Point(10, 10);
Controls.Add(pictureBox);
}
private void Timer_Tick(object sender, EventArgs e)
{
// 每次定时器触发时,将PictureBox的位置向左移动一定距离
pictureBox.Left -= 5;
// 当PictureBox完全移出窗口时,将其重新放置到窗口右侧
if (pictureBox.Right < 0)
{
pictureBox.Left = Width;
}
}
}
}
```
相关推荐
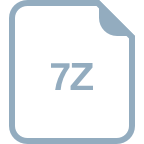














