android studio身份证录入,请用Java语言给出我有一个完整的代码
时间: 2024-10-21 20:18:42 浏览: 35
Android Studio中集成身份证识别功能通常需要借助第三方库,如ZBar条码扫描库、身份证OCR识别库等。这些库可以帮助读取图片中的身份证信息,但请注意,直接在Android Studio内处理身份证识别可能会涉及到隐私问题,因此一般建议在服务器端完成此类敏感操作。
如果你想要在Java中使用OCR(Optical Character Recognition,光学字符识别)技术识别身份证,你需要先在服务器上安装OCR引擎,如Tesseract OCR,然后通过网络请求获取结果。这里仅提供一个简化版的伪代码示例,实际项目中还需要考虑权限、数据传输安全等因素:
```java
// 导入必要的库,例如HttpClient用于网络请求,TessBaseAPI用于OCR识别
import org.apache.http.HttpEntity;
import net.sourceforge.tess4j.*;
public class IDCardRecognizer {
private static final String API_URL = "http://your_server_url/ocr"; // 替换为你服务器的地址
public static String recognize(String imageBase64) throws Exception {
// 创建HttpClient实例并发送POST请求
HttpClient httpClient = new DefaultHttpClient();
HttpPost httpPost = new HttpPost(API_URL);
StringEntity stringEntity = new StringEntity(imageBase64);
httpPost.setEntity(stringEntity);
// 设置编码
httpPost.setHeader("Content-Type", "application/x-www-form-urlencoded");
// 发送请求并获取响应
HttpResponse httpResponse = httpClient.execute(httpPost);
HttpEntity responseEntity = httpResponse.getEntity();
if (responseEntity != null) {
// 从服务器获取到的Base64解码后的身份证文本
byte[] resultBytes = EntityUtils.toByteArray(responseEntity);
String idCardText = new String(resultBytes, StandardCharsets.UTF_8);
// 关闭连接
responseEntity.consumeContent();
httpClient.getConnectionManager().shutdown();
return idCardText; // 返回识别的结果
} else {
throw new RuntimeException("Failed to get response from server.");
}
}
}
// 调用方法示例
try {
String imageData = "base64_encoded_image_data"; // 需要替换为实际的Base64编码图像数据
String idCardInfo = IDCardRecognizer.recognize(imageData);
System.out.println("身份证信息: " + idCardInfo);
} catch (Exception e) {
e.printStackTrace();
}
```
阅读全文
相关推荐
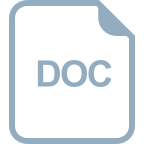
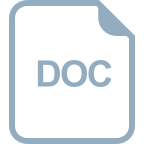
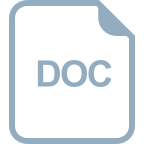
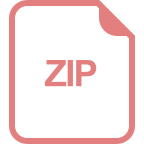
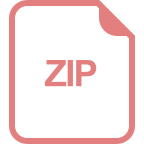
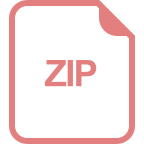
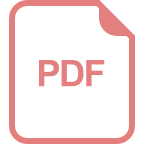
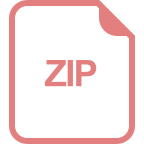
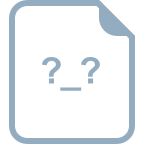
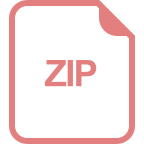
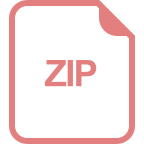
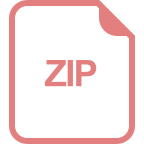
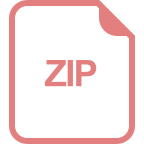
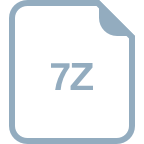
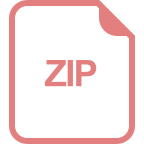