使用c++读入n值及n个整数,建立顺序表并遍历输出
时间: 2024-10-24 18:11:47 浏览: 24
在C++中,你可以通过`cin`输入流来读取用户提供的数值,然后使用动态数组或容器如`std::vector`来创建一个顺序表存储这些整数。以下是简单的步骤:
1. 首先,包含必要的头文件:
```cpp
#include <iostream>
#include <vector>
using namespace std;
```
2. 创建一个函数来读取输入并构建顺序表:
```cpp
void readAndBuildList(int& n) {
vector<int> sequence; // 顺序表
cout << "请输入整数的数量 (n): ";
cin >> n;
for (int i = 0; i < n; ++i) {
int number;
cout << "请输入第" << (i+1) << "个整数: ";
cin >> number;
sequence.push_back(number); // 将整数添加到列表中
}
}
```
3. 然后,你可以编写一个函数来遍历并输出这个顺序表:
```cpp
void printList(const vector<int>& sequence) {
cout << "顺序表内容为: ";
for (const auto& num : sequence) {
cout << num << " ";
}
cout << endl;
}
```
4. 最后,在主函数中调用这两个函数:
```cpp
int main() {
int n;
readAndBuildList(n);
printList(sequence); // 输出顺序表
return 0;
}
```
当运行这个程序时,它会提示用户输入数字,并显示相应的顺序列表。
阅读全文
相关推荐
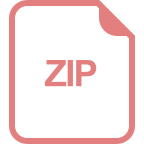
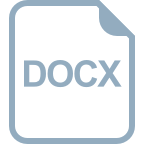
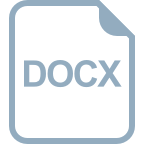




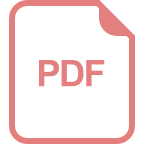









