uniapp vue3全局自定义组件
时间: 2023-07-17 19:01:16 浏览: 239
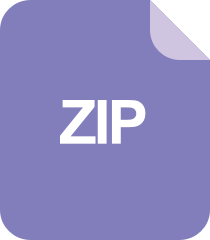
vue3 封装一个全局通用弹窗提示组件(利用js调用 类似于element)

对于UniApp中使用Vue 3全局自定义组件,可以按照以下步骤进行操作:
1. 在项目根目录下创建一个components文件夹,用于存放全局自定义组件。
2. 在components文件夹下创建一个新的.vue文件,作为你的自定义组件。
3. 在这个.vue文件中,定义你的组件的模板、样式和逻辑。
4. 在main.js(或者其他入口文件)中引入这个组件,并通过Vue.component()方法将其注册为全局组件。例如:
```javascript
import YourComponent from '@/components/YourComponent.vue'
Vue.component('your-component', YourComponent)
```
5. 在需要使用这个全局组件的页面中,直接使用该组件的标签名即可,无需再进行引入。例如:
```html
<template>
<div>
<your-component></your-component>
</div>
</template>
```
这样,你就可以在整个UniApp项目中使用这个全局自定义组件了。记得在使用时根据需要传递相应的props或者监听相应的事件。
希望能对你有所帮助!如有更多问题,请继续提问。
阅读全文
相关推荐
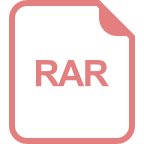
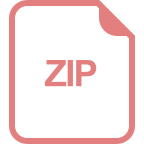















