python文字排版工具要求实现删除空格,英文标点替换,英文单词大写的功能代码
时间: 2024-10-17 11:06:24 浏览: 30
在Python中,你可以使用内置的字符串方法和正则表达式库`re`来实现文本处理功能,如删除空格、替换英文标点并转换成大写。下面是一个简单的示例代码:
```python
import re
def text_formatting(text):
# 删除空格
no_spaces = text.replace(" ", "")
# 替换英文标点,这里使用了全角字符范围(适用于ASCII字符集)
punctuation_replacement = re.sub(r'[^\w\s]', '', no_spaces)
# 将所有英文单词转为大写
capitalized_words = punctuation_replacement.upper()
return capitalized_words
# 使用示例
input_text = "Hello, World! This is a test."
formatted_text = text_formatting(input_text)
print(formatted_text)
```
相关问题
写一个python程序,输入一个由英文字符、数字、空格和英文标点符号组成的字符串,要求将其切分为单词,去掉所有的非英文字母,然后将单词全部转换为小写,在按照字典序升序排列,每个单词用空格分隔。
可以使用正则表达式来去除非英文字母,然后使用split函数来切分字符串。最后使用sorted函数按照字典序升序排列单词。
以下是一个示例程序:
```python
import re
# 输入字符串
s = input("请输入字符串:")
# 去除非英文字母,转换为小写,切分为单词
words = re.findall(r'[a-zA-Z]+', s)
words = [word.lower() for word in words]
# 按照字典序升序排列单词
words = sorted(words)
# 输出结果
print(" ".join(words))
```
示例输入:
```
Hello World! This is a Test. 1234
```
示例输出:
```
a hello is test this world
```
Python将一段话中的标点替换成空格、
可以使用Python中的字符串替换函数(replace),代码如下:
```python
text = "Hello, World! This is a sentence."
clean_text = text.replace(",", " ").replace(".", " ").replace("!", " ") # 将逗号、句号、感叹号都替换成空格
print(clean_text)
```
输出结果为:
```
Hello World This is a sentence
```
阅读全文
相关推荐
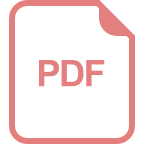
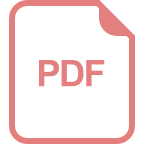
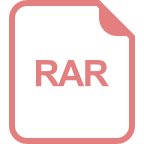












