flutter android 拍照上传图片
时间: 2024-11-12 13:15:11 浏览: 6
在Flutter中,如果你想要在Android应用中实现拍照并上传图片的功能,可以按照以下步骤操作:
1. **添加依赖**:首先,你需要在`pubspec.yaml`文件中添加`image_picker`和`path_provider`库,这两个库分别用于选择图片和处理路径:
```yaml
dependencies:
image_picker: ^0.8.4+4
path_provider: ^2.0.5
```
然后运行`flutter pub get`安装。
2. **导入库**:在需要使用的地方导入这两个库:
```dart
import 'package:image_picker/image_picker.dart';
import 'package:path_provider/path_provider.dart';
```
3. **权限请求**:在`AndroidManifest.xml`中添加相机和存储权限:
```xml
<uses-permission android:name="android.permission.CAMERA" />
<uses-permission android:name="android.permission.WRITE_EXTERNAL_STORAGE" />
```
4. **拍照功能**:创建一个函数,调用`ImagePicker`的`getImageSource`方法来打开相机或相册:
```dart
Future<void> pickImage() async {
final picker = ImagePicker();
final pickedFile = await picker.getImage(source: ImageSource.camera);
if (pickedFile != null) {
// 处理获取到的图片
handlePickedImage(pickedFile);
} else {
print('No image selected.');
}
}
Future<void> handlePickedImage(FileImage file) async {
// 使用path_provider获取文件路径
final Directory? appDocDir = await getApplicationDocumentsDirectory();
final String imagePath = '${appDocDir!.path}/image.jpg';
await file.copy(imagePath);
// 这里可以进一步上传图片,比如通过网络请求将路径传给服务器
uploadImage(imagePath);
}
```
5. **上传图片**:在`uploadImage`函数中,你可以使用第三方库如`http`或`dio`来发送HTTP请求,将图片路径作为附件上传到服务器:
```dart
Future<void> uploadImage(String imagePath) async {
final response = await http.post(
Uri.parse('https://your-api-url/upload'),
headers: {'Content-Type': 'multipart/form-data'},
body: multipartBody(imagePath),
);
if (response.statusCode == 200) {
// 图片上传成功,处理响应数据
print('Image uploaded successfully');
} else {
print('Failed to upload image: ${response.statusCode}');
}
}
MultipartFile multipartBody(String imagePath) {
return MultipartFile.fromPath('image', imagePath);
}
```
6. **处理错误**:记得处理可能出现的异常,比如文件读取失败、网络连接问题等。
阅读全文
相关推荐
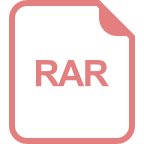
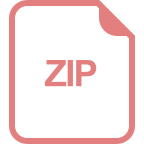
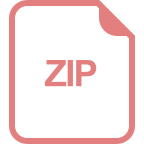

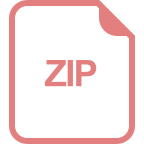

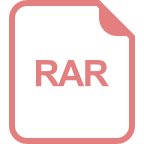
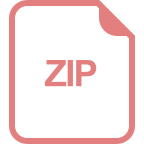
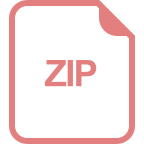
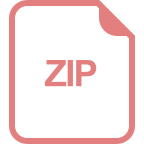
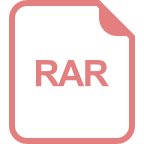
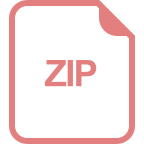
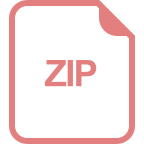
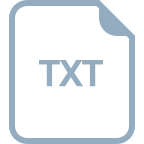
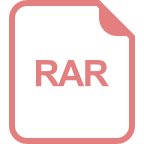
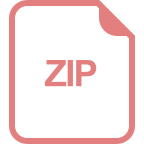
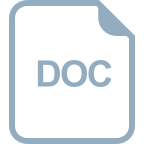
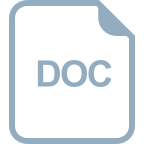
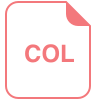