opencvsharp 查找最大四边形
时间: 2023-07-29 09:08:37 浏览: 98
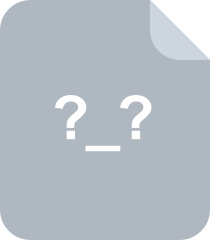
opencv 图像边框寻找
你可以使用OpenCvSharp来查找图像中的最大四边形。以下是一个示例代码,展示了如何使用OpenCvSharp进行最大四边形的查找:
```csharp
using OpenCvSharp;
using OpenCvSharp.Extensions;
// 读取图像
Mat image = Cv2.ImRead("image.jpg", ImreadModes.Color);
// 将图像转换为灰度图像
Mat gray = new Mat();
Cv2.CvtColor(image, gray, ColorConversionCodes.BGR2GRAY);
// 对灰度图像进行二值化处理
Mat binary = new Mat();
Cv2.Threshold(gray, binary, 0, 255, ThresholdTypes.Binary | ThresholdTypes.Otsu);
// 查找轮廓
Point[][] contours;
HierarchyIndex[] hierarchy;
Cv2.FindContours(binary, out contours, out hierarchy, RetrievalModes.External, ContourApproximationModes.ApproxSimple);
// 找到最大的四边形
double maxArea = 0;
RotatedRect maxRect = new RotatedRect();
foreach (var contour in contours)
{
var approx = Cv2.ApproxPolyDP(contour, Cv2.ArcLength(contour, true) * 0.02, true);
if (approx.Length == 4 && Cv2.IsContourConvex(approx))
{
double area = Cv2.ContourArea(approx);
if (area > maxArea)
{
maxArea = area;
maxRect = Cv2.MinAreaRect(approx);
}
}
}
// 在图像上绘制最大四边形
Cv2.DrawContours(image, new Point[][] { maxRect.Points() }, -1, Scalar.Red, 2);
// 显示结果
Cv2.ImShow("Max Quadrilateral", image);
Cv2.WaitKey(0);
Cv2.DestroyAllWindows();
```
上述代码中,首先读取图像并将其转换为灰度图像。然后对灰度图像进行二值化处理,以便查找轮廓。使用`FindContours`函数找到图像中的所有轮廓,并通过`ApproxPolyDP`函数对每个轮廓进行多边形逼近,筛选出四边形轮廓。最后,通过计算四边形的面积,找到最大的四边形,并在原图上绘制出来。
请确保你已经安装了OpenCvSharp库,并替换代码中的图像路径为你自己的图像路径。希望对你有帮助!如果有任何问题,请随时提问。
阅读全文
相关推荐
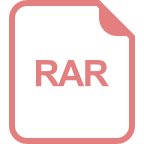

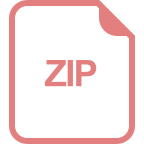
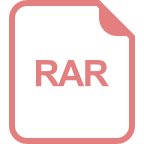
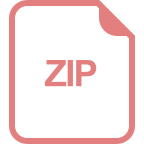
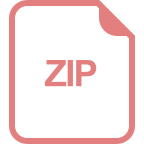
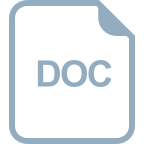
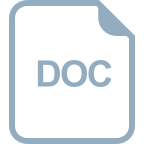
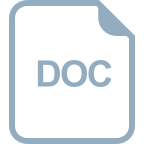
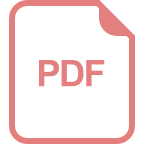
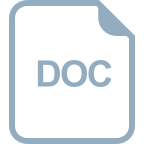
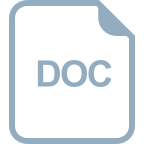
