ollama python 原生实现多agent交互
时间: 2024-08-09 13:01:25 浏览: 83
`ollama`是一个基于Python构建的框架,专用于模拟、协调和分析多智能体系统(Multi-Agent Systems,MAS)。在`ollama`中实现多Agent交互主要包括以下几个关键方面:
### 1. Agent设计
首先,你需要创建一个或多个Agent类,并在其中定义每个Agent的行为逻辑。这通常涉及到初始化函数(__init__),以及执行特定任务的函数。
```python
class MyAgent(ollama.Agent):
def __init__(self):
super().__init__()
self.strategy = "greedy"
def decide(self, state):
# 根据当前状态做出决策
if self.strategy == "greedy":
action = self.greedy_strategy(state)
elif self.strategy == "cooperative":
action = self.cooperative_strategy(state)
return action
```
### 2. 环境设定
环境(Environment)描述了所有Agent活动的场景,包括初始条件、规则、奖励机制等。
```python
class GameEnvironment(ollama.Environment):
def __init__(self):
super().__init__()
self.initialize()
self.reward = 0
def step(self):
for agent in self.agents:
state = self.observe(agent)
action = agent.decide(state)
result = self.execute_action(action)
reward = self.compute_reward(result)
agent.receive_feedback(reward)
self.update_state()
self.check_termination()
```
### 3. 协同与通信
在多Agent系统中,协同工作至关重要。Agent之间可以共享信息,通过消息传递进行交流。`ollama`提供了一种结构化的方式来实现这一点。
```python
def communicate(self, message):
for other_agent in self.environment.agents:
if other_agent is not self:
other_agent.receive_message(message)
# 使用示例
my_agent.communicate({"type": "info", "message": "Hello from agent A"})
```
### 相关问题:
1. `ollama`如何支持复杂多Agent系统的协作?
2. 实现多Agent系统时需要注意哪些挑战和解决方案?
3. `ollama`是否适用于所有类型的多Agent系统需求?
---
以上是使用Python原生实现多Agent交互的基本概述,`ollama`提供了一个更高级别的抽象层,可以帮助简化多Agent系统的设计和实现过程。如果你有兴趣深入了解具体的API使用、案例研究或是进一步的功能特性,请随时提问。
相关推荐
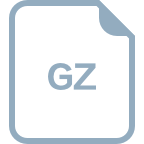
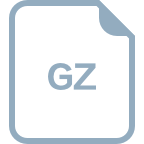
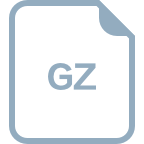














