经过完善后,最后所有的代码是哪些
时间: 2024-11-20 20:40:01 浏览: 10
经过完善后的`DestroyByContact` 和 `GameController` 类的代码会包含上述提到的UI提示和按键交互的部分。以下是合并后的完整代码:
```csharp
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.UI;
using TMPro;
using UnityEngine.SceneManagement;
public class DestroyByContact : MonoBehaviour
{
public GameObject explosion;
public GameObject playerExplosion;
public GameObject gameOverMessage; // 新增的UI游戏结束提示
private GameController gameController;
void Start()
{
GameObject gameControllerObject = GameObject.FindWithTag("GameController");
if (gameControllerObject != null)
{
gameController = gameControllerObject.GetComponent<GameController>();
}
if (gameController == null)
{
Debug.Log("Cannot find 'GameController' script");
}
}
void OnTriggerEnter(Collider other)
{
if (other.tag == "Boundary")
{
return;
}
Instantiate(explosion, transform.position, transform.rotation);
if (other.tag == "Player")
{
Instantiate(playerExplosion, other.transform.position, other.transform.rotation);
if (gameController != null)
{
gameController.GameOver();
}
}
Destroy(other.gameObject);
Destroy(gameObject);
}
public void OnEscapeKeyDown()
{
// 增加对 'Esc' 键的监听,可以在Update()里检查
if (Input.GetKeyDown(KeyCode.Escape))
{
gameController.RestartGame(); // 假设GameController有一个RestartGame方法
}
}
}
public class GameController : MonoBehaviour
{
public GameObject hazard;
public Vector3 spawnValues;
public int hazardCount;
public float spawnWait;
public float startWait;
public float waveWait;
private int score = 0;
public TextMeshProUGUI scoreText;
public TextMeshProUGUI gameOverText;
public TextMeshProUGUI helpText;
private bool gameOver;
private float fadeAlpha = 0; // 透明度值
private float fadeSpeed = 2f; // 淡入速度
public GameObject gameOverMessageDisplay; // 原有的提示UI
void Start()
{
gameOver = false;
gameOverText.text = " ";
helpText.text = " ";
StartCoroutine(SpawnWaves());
}
void Update()
{
if (Input.GetKeyDown(KeyCode.R))
{
int currentSceneIndex = SceneManager.GetActiveScene().buildIndex;
SceneManager.LoadScene(currentSceneIndex);
}
// 添加 Esc 键监听
if (Input.GetKeyDown(KeyCode.Escape))
{
OnEscapeKeyDown();
}
}
public void GameOver()
{
gameOver = true;
gameOverText.text = "Game Over!";
helpText.text = " Press 'R' to Restart or 'Esc' to Continue...";
// 显示游戏结束提示
gameOverMessageDisplay.SetActive(true);
StartCoroutine(FadeOutMessage());
}
public void RestartGame()
{
gameOver = false;
gameOverText.text = " ";
helpText.text = " Press 'R' to Restart or 'Esc' to Continue...";
// 清除游戏结束提示
gameOverMessageDisplay.SetActive(false);
// 渲染新的游戏界面或加载新场景
}
IEnumerator FadeOutMessage()
{
float fadeInTime = 1f; // 淡出时间,单位秒
while (fadeAlpha < 1)
{
fadeAlpha = Mathf.Lerp(fadeAlpha, 1, Time.deltaTime / fadeInTime);
gameOverText.color = new Color(gameOverText.color.r, gameOverText.color.g, gameOverText.color.b, fadeAlpha);
gameOverMessageDisplay.SetActive(false); // 隐藏提示UI
yield return null;
}
// 清除提示文本
gameOverText.text = "";
gameOverMessageDisplay.SetActive(false);
}
public void addScore(int value)
{
score += value;
UpdateScore();
}
private void UpdateScore()
{
scoreText.text = "Score: " + score.ToString();
}
IEnumerator SpawnWaves()
{
yield return new WaitForSeconds(startWait);
while (true)
{
for (int i = 0; i < hazardCount; i++)
{
Vector3 spawnPosition = new Vector3(Random.Range(-spawnValues.x, spawnValues.x), spawnValues.y, spawnValues.z);
Quaternion spawnRotation = Quaternion.identity;
Instantiate(hazard, spawnPosition, spawnRotation);
yield return new WaitForSeconds(spawnWait);
}
yield return new WaitForSeconds(waveWait);
if (gameOver)
{
break;
}
}
}
}
```
阅读全文
相关推荐
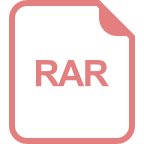
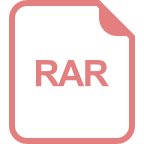
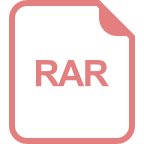
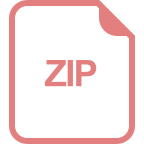
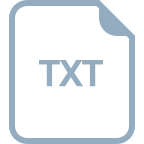
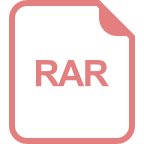
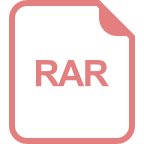
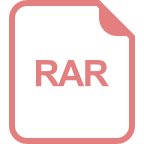
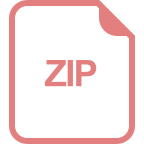
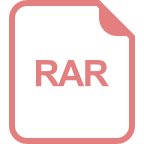
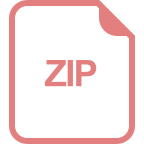
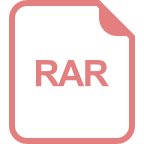
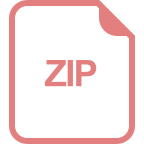
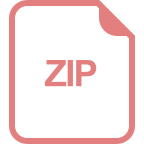
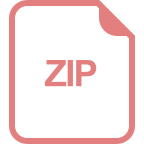
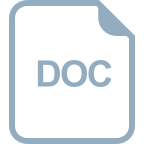
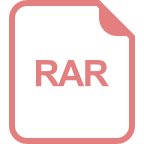
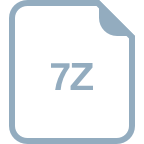