tf/transform_broadcaster.h 文件路径
时间: 2024-05-10 21:13:21 浏览: 115
tf/transform_broadcaster.h 文件路径在ROS中是:
/opt/ros/<ros_version>/include/tf/transform_broadcaster.h
其中<ros_version>为你所使用的ROS版本,例如在ROS Noetic中,路径应为:
/opt/ros/noetic/include/tf/transform_broadcaster.h
该文件包含了tf库中用于发布变换的TransformBroadcaster类的定义,可以用于发布不同坐标系之间的变换信息。
相关问题
<launch> <param name ="/use_sim_time" value ="false"/> <arg name ="urdf_file" default ="$(find xacro)/xacro '$(find smartcar)/urdf/smartcar.urdf.xacro'"/> <arg name ="gui" default="false"/> <param name ="robot_description" command=" $(arg urdf_file)"/> <param name ="use_gui" value ="$(arg gui)"/> <node name="arbotix" pkg="arbotix_python" type="arbotix_driver" output="screen"> <rosparam file="$(find smartcar)/config/smartcar_arbotix.yaml" command="load"/> <param name="sim" value="true" /> </node> <node name =" join _state_publisher_gui" pkg ="joint_state_publisher_gui" type ="joint_state_publisher_gui"></node > <node name =" robot_state_publisher " pkg ="robot_state_publisher" type ="robot_state_publisher"> <param name=" publish_frequency" type ="double" value ="20.0"/> </node> <node pkg ="tf" type ="static_transform_publisher" name ="odom_left_wheel_broadcaster" args="0 0 0 0 0 0 /base_link /left_front_link 100" /> <node pkg ="tf" type ="static_transform_publisher" name ="odom_right_wheel_broadcaster" args="0 0 0 0 0 0 /base_link /right_front_link 100" /> <node name ="rviz" pkg ="rviz" type ="rviz" args ="-d $(find smartcar)/config/smartcar_urdf.rviz" required="true"/> </launch>
根据你提供的launch文件,你的URDF文件路径有问题。在第3行的`<arg>`标签中,你将`default`参数设置为以下值:
```
$(find xacro)/xacro '$(find smartcar)/urdf/smartcar.urdf.xacro'
```
这个值应该是一个有效的命令行字符串,用于加载URDF文件。然而,这个命令行字符串似乎不正确,因为它包含了两个`$(find)`占位符。正确的命令行字符串应该是:
```
$(find xacro)/xacro '$(find smartcar)/urdf/smartcar.urdf.xacro'
```
这个命令行字符串使用`xacro`工具来解析你的URDF文件,并将结果作为URDF文件的路径。请注意,你需要确保`smartcar.urdf.xacro`文件位于`smartcar`软件包的`urdf`目录下。
如果你仍然遇到问题,请检查命令行输出和启动日志,以获取更多信息。
C++程序调用robot_localization和amcl功能包实现激光雷达和IMU定位
要在C++程序中调用robot_localization和AMCL功能包实现激光雷达和IMU定位,需要进行以下步骤:
1. 首先,确保你已经安装了ROS,并且已经创建了一个ROS包来存放你的C++程序。
2. 通过在命令行中输入以下命令安装robot_localization和AMCL功能包:
```
sudo apt-get install ros-<your-ros-version>-robot-localization ros-<your-ros-version>-amcl
```
其中,`<your-ros-version>`是你正在使用的ROS版本,例如`melodic`或`noetic`。
3. 然后,在你的C++程序中包含以下头文件:
```
#include <ros/ros.h>
#include <robot_localization/navsat_conversions.h>
#include <robot_localization/ros_filter_utilities.h>
#include <robot_localization/srv/set_pose.h>
#include <geometry_msgs/PoseWithCovarianceStamped.h>
#include <sensor_msgs/Imu.h>
#include <sensor_msgs/NavSatFix.h>
#include <tf2/LinearMath/Quaternion.h>
#include <tf2_ros/transform_listener.h>
#include <tf2_geometry_msgs/tf2_geometry_msgs.h>
#include <tf2_ros/buffer.h>
#include <tf/transform_listener.h>
#include <tf/transform_datatypes.h>
#include <tf_conversions/tf_eigen.h>
#include <tf/transform_broadcaster.h>
#include <tf/LinearMath/Matrix3x3.h>
#include <string>
#include <vector>
#include <iostream>
#include <fstream>
#include <cmath>
#include <Eigen/Dense>
#include <nav_msgs/Odometry.h>
#include <geometry_msgs/Pose.h>
#include <geometry_msgs/PoseStamped.h>
#include <geometry_msgs/Twist.h>
#include <geometry_msgs/TwistStamped.h>
#include <std_msgs/Float64.h>
#include <std_msgs/Bool.h>
#include <std_msgs/String.h>
```
4. 然后,在你的代码中创建ROS节点,并订阅激光雷达和IMU的数据,以及发布机器人的位置信息。
```
int main(int argc, char** argv)
{
// 初始化ROS节点
ros::init(argc, argv, "my_robot_localization_node");
ros::NodeHandle nh;
// 创建订阅器
ros::Subscriber imu_sub = nh.subscribe("/imu", 1000, imuCallback);
ros::Subscriber laser_sub = nh.subscribe("/laser_scan", 1000, laserCallback);
// 创建发布器
ros::Publisher pose_pub = nh.advertise<nav_msgs::Odometry>("/robot_pose", 1000);
// 循环读取数据并发布位置信息
while(ros::ok())
{
// 在这里进行数据融合的处理
// 发布位置信息
pose_pub.publish(odom);
// 延时一段时间
ros::Duration(0.1).sleep();
}
return 0;
}
```
5. 在`imuCallback()`和`laserCallback()`函数中,将接收到的IMU和激光雷达数据保存到ROS消息中,然后在主循环中进行数据融合的处理,并将融合后的位置信息发布到`/robot_pose`话题中。
```
void imuCallback(const sensor_msgs::Imu::ConstPtr& msg)
{
// 将IMU数据保存到ROS消息中
imu_data = *msg;
}
void laserCallback(const sensor_msgs::LaserScan::ConstPtr& msg)
{
// 将激光雷达数据保存到ROS消息中
laser_data = *msg;
}
```
6. 最后,编译你的C++程序并运行它:
```
cd <your-catkin-workspace>/src
catkin_make
source devel/setup.bash
rosrun <your-ros-package> <your-cpp-program>
```
其中,`<your-catkin-workspace>`是你的Catkin工作空间的路径,`<your-ros-package>`是你存放C++程序的ROS包的名称,`<your-cpp-program>`是你的C++程序的名称。
阅读全文
相关推荐
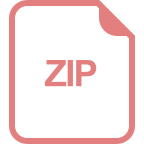
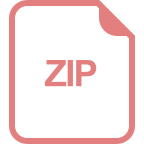
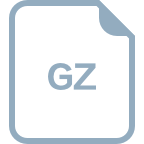
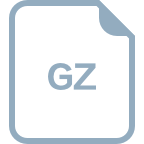
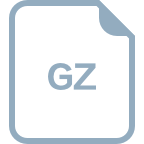
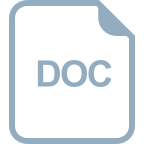
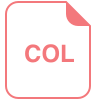
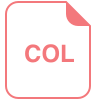
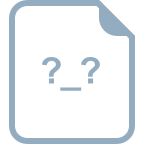
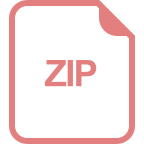
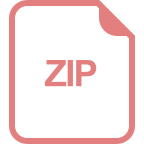
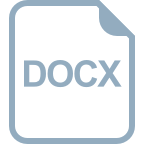
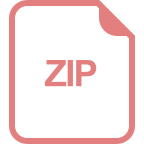
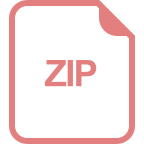
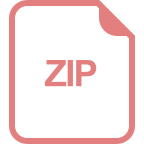