*** 在Linux环境下编写一个应用程序B,简要说明此程序能够体现操作系统的并发性、异步性、共享性和持久性。(基于C或Rust语言)
时间: 2024-09-23 15:14:19 浏览: 74
在Linux环境下,编写一个名为B的应用程序,我们可以选择C语言或Rust语言来体现操作系统的并发性、异步性、共享性和持久性。
1. **并发性**:可以使用C的`pthread`库或者Rust的`std::thread`创建并管理线程,通过同时运行多个任务来展示并发。比如创建两个互斥的任务,一个负责读取文件,另一个负责处理数据,它们在时间上并行运行,提高效率。
```c
#include <pthread.h>
void *task1(void *arg);
void *task2(void *arg);
int main() {
pthread_t thread1, thread2;
pthread_create(&thread1, NULL, task1, NULL);
pthread_create(&thread2, NULL, task2, NULL);
// 等待所有任务完成
pthread_join(thread1, NULL);
pthread_join(thread2, NULL);
}
// 示例任务函数
void *task1(void *arg) {
// 执行读取文件的操作
}
```
2. **异步性**:利用C的`select`系统调用或Rust的`tokio`库可以实现非阻塞I/O,允许程序在等待IO操作完成时继续执行其他任务。这样,当数据可用时,回调函数会被自动调用,体现了异步编程模型。
```c
#include <sys/select.h>
int main() {
int fd[2];
if (pipe(fd) == -1) { ... }
select(fd[0], NULL, &fd[1], NULL, NULL);
// 当数据准备好时,此处将执行相应的处理
}
```
3. **共享性**:C的全局变量或Rust的静态变量可以在多个函数之间共享,通过指针或引用访问。通过锁(如C的`mutex`或Rust的`Mutex`)保证对共享资源的安全访问。
4. **持久性**:使用文件操作库(如C的`fcntl`或Rust的`fs`)创建、打开和保存数据到磁盘,使得数据即使程序结束也能持久存在。例如:
```c
#include <fcntl.h>
struct Data {
// 数据结构...
};
int main() {
struct Data data;
int fd = open("data.txt", O_CREAT | O_RDWR, 0644);
// 写入数据
write(fd, &data, sizeof(data));
close(fd);
}
```
阅读全文
相关推荐
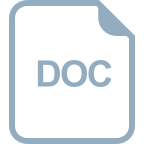






