c++写一段公寓缴费的功能
时间: 2024-09-21 20:11:38 浏览: 41
在C++中编写一段简单的公寓缴费功能,我们可以创建一个`ApartmentBill`类,包含用户信息、费用以及支付方法等功能。下面是一个简化的例子:
```cpp
#include <iostream>
#include <string>
class User {
public:
std::string name;
int apartmentID;
// 构造函数
User(std::string n, int a) : name(n), apartmentID(a) {}
};
class ApartmentBill {
private:
User user;
double monthlyFee;
bool isPaid;
public:
// 构造函数
ApartmentBill(const User& u, double f) : user(u), monthlyFee(f), isPaid(false) {}
// 缴费方法
void payBill() {
std::cout << "用户 " << user.name << " 的公寓 (" << user.apartmentID << ") 本月费用为 " << monthlyFee << " 元。\n";
if (monthlyFee > 0) {
std::cout << "请输入支付金额:";
double payment;
std::cin >> payment;
if (payment >= monthlyFee) {
isPaid = true;
std::cout << "已成功缴纳,剩余金额为 0。\n";
} else {
std::cout << "支付金额不足!\n";
}
} else {
std::cout << "当前无费用需缴纳。\n";
}
}
// 检查是否已支付
bool isPaidStatus() const {
return isPaid;
}
};
int main() {
User user("张三", 101);
ApartmentBill bill(user, 1500);
bill.payBill();
if (bill.isPaidStatus()) {
std::cout << "账单状态: 已支付\n";
} else {
std::cout << "账单状态: 未支付\n";
}
return 0;
}
```
在这个示例中,我们首先定义了用户和公寓账单两个类,然后实现了基本的缴费流程,包括提示输入费用、检查付款金额是否足够等。`main()`函数中实例化了一个用户和一个账单,并展示了如何使用。
阅读全文
相关推荐
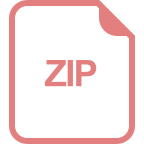
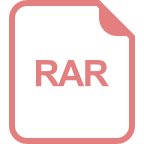
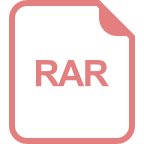
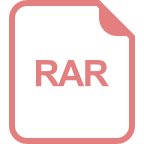
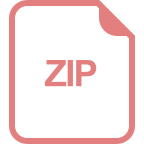
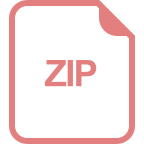
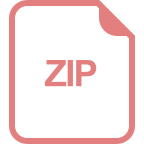
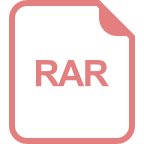
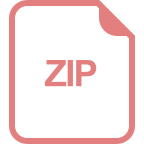
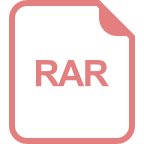
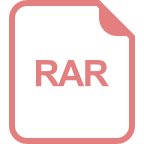
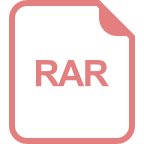
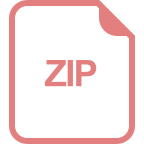
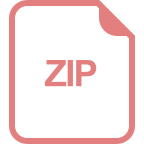
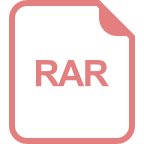
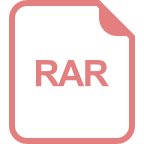