如何用汇编语言(包括子程序和嵌套结构)编写一个MASM程序来检测输入字符串中是否包含'abc'这个子串?
时间: 2024-12-12 07:27:52 浏览: 17
使用Microsoft Assembler (MASM) 编写一个检测输入字符串中是否包含 'abc' 子串的程序,我们可以采用递归方法(通过子程序实现)或者循环遍历。这里以递归为例,因为它能展示子程序和嵌套结构的概念:
```assembly
; 宏定义子程序,接收字符串地址和子串地址作为参数
macro contains_substring(input, substring)
push ebp
mov ebp, esp
mov esi, [ebp + 8] ; 保存输入字符串地址
mov edi, [ebp + 12] ; 保存子串地址
call strcmp_recursive ; 调用子程序进行比较
pop ebp
ret
contains_substring ENDM
; 主程序入口点
section .data
input db 'your_input_string', 0
ABC db 'abc', 0
result db 1 dup(?)
section .text
global _start
_start:
; 判断输入字符串是否包含 "abc"
push input
push ABC
call contains_substring
cmp eax, 0 ; 如果相等(等于0表示相匹配),则结果为1(存在)
je found
xor eax, eax ; 不匹配,设置结果为0
mov [result], al
found:
; 输出或处理结果...
; ...
strcmp_recursive proc
; 检查当前字符是否相等,不等则结束递归
cmp byte [esi], byte [edi]
jne not_match
; 递归进入下一位,直到遍历完子串
add esi, 1
add edi, 1
cmp edi, ebx ; ebx用于临时存储子串长度
jl recursive_call
; 匹配,返回0
xor eax, eax
jmp done
not_match:
; 不匹配,返回-1
sub eax, 1
recursive_call:
push eax
call strcmp_recursive
add esp, 4
cmp eax, -1
je not_match ; 如果递归中未找到,继续查找
done:
ret
strcmp_recursive endp
; 结束主程序
xor eax, eax
pop ebx
add esp, 4
mov eax, [result]
; 程序退出...
```
在这个示例中,`strcmp_recursive` 函数作为子程序实现字符串比较,`contains_substring` 是一个宏定义的外部函数,内部调用了子程序。如果在 `contains_substring` 中找到 'abc',`eax` 将是0,反之则是1(表示不存在)。
阅读全文
相关推荐
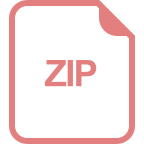
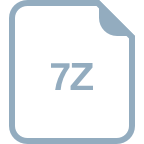

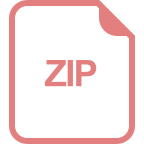
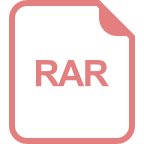
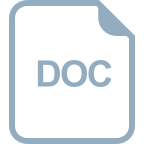
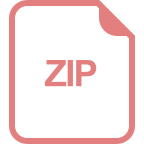
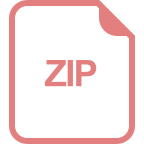
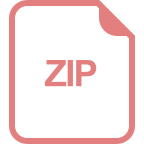
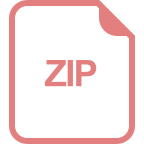
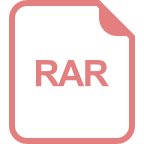
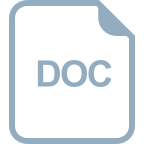
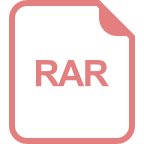
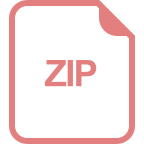
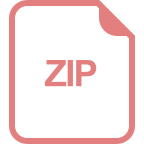
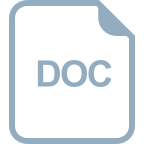
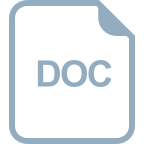