定义一个学生bean类,封装学号、姓名、生日、专业、成绩五个属性,在JSP页面通过表单提交一个学生的相关信息存入bean对象中,然后获取这个bean的属性值输出。
时间: 2024-11-27 07:08:21 浏览: 10
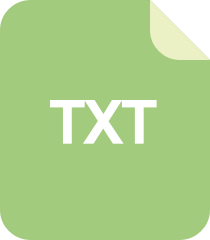
jsp九个内置对象 六个基本动作 三个指令元素

在Java Web开发中,我们可以创建一个简单的StudentBean类来封装上述属性。首先,定义一个名为Student的Java类:
```java
import java.util.Date;
public class Student {
private String studentID;
private String name;
private Date birthday;
private String major;
private double score;
// 构造函数
public Student(String studentID, String name, Date birthday, String major, double score) {
this.studentID = studentID;
this.name = name;
this.birthday = birthday;
this.major = major;
this.score = score;
}
// 获取和设置属性的方法
public String getStudentID() { return studentID; }
public void setStudentID(String studentID) { this.studentID = studentID; }
public String getName() { return name; }
public void setName(String name) { this.name = name; }
public Date getBirthday() { return birthday; }
public void setBirthday(Date birthday) { this.birthday = birthday; }
public String getMajor() { return major; }
public void setMajor(String major) { this.major = major; }
public double getScore() { return score; }
public void setScore(double score) { this.score = score; }
@Override
public String toString() {
return "Student{" +
"studentID='" + studentID + '\'' +
", name='" + name + '\'' +
", birthday=" + birthday +
", major='" + major + '\'' +
", score=" + score +
'}';
}
}
```
接下来,我们会在JSP页面上创建一个HTML表单,用于输入学生的数据并将其绑定到StudentBean实例:
```jsp
<form action="submitStudent" method="post">
<label for="studentID">学号:</label>
<input type="text" id="studentID" name="studentID"><br><br>
<label for="name">姓名:</label>
<input type="text" id="name" name="name"><br><br>
<label for="birthday">生日:</label>
<input type="date" id="birthday" name="birthday"><br><br>
<label for="major">专业:</label>
<input type="text" id="major" name="major"><br><br>
<label for="score">成绩:</label>
<input type="number" id="score" name="score"><br><br>
<input type="submit" value="提交">
</form>
<%-- 显示学生信息 --%>
<c:if test="${not empty student}">
<h2>学生信息:</h2>
<p>${student.studentID}</p>
<p>${student.name}</p>
<p>生日: ${student.birthday}</p>
<p>专业: ${student.major}</p>
<p>成绩: ${student.score}</p>
</c:if>
```
最后,我们需要在Servlet或Controller(如果使用Spring MVC)中处理表单提交,将数据注入到StudentBean实例,并可能保存到数据库或返回响应给前端。
阅读全文
相关推荐
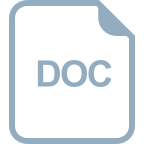
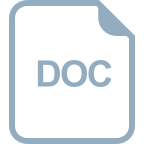
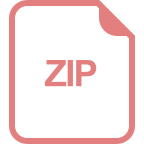
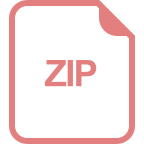
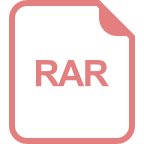
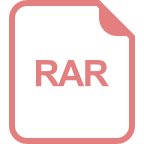
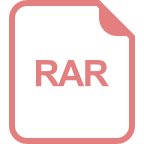
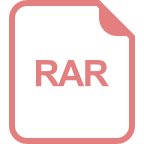
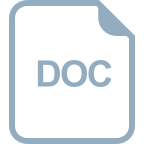
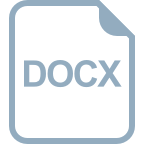
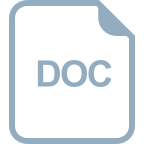
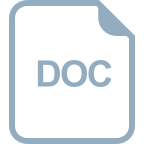
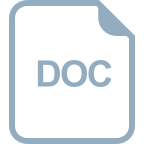
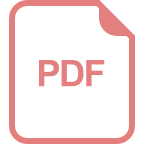
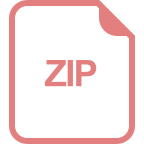
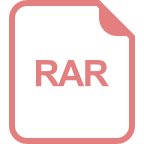
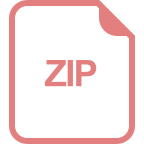
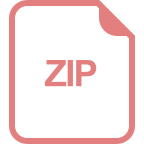