将该代码在Python当中表示
时间: 2024-12-22 21:18:54 浏览: 3
将给定的MATLAB代码转换为Python代码需要使用相应的Python库(如NumPy)来处理数组和数学运算。以下是该代码的Python版本:
```python
import numpy as np
def CPO(Pop_size, Tmax, lb, ub, dim, fobj):
# Definitions
Conv_curve = np.zeros(Tmax)
ub = ub * np.ones(dim)
lb = lb * np.ones(dim)
# Controlling parameters
N = Pop_size # Initial population size
N_min = int(0.8 * Pop_size) # Minimum population size
T = 2 # Number of cycles
alpha = 0.2 # Convergence rate
Tf = 0.8 # Tradeoff percentage between the third and fourth defense mechanisms
# Initialization
X = initialization(Pop_size, dim, ub, lb)
t = 0 # Function evaluation counter
# Evaluation
fitness = np.array([fobj(X[i, :]) for i in range(Pop_size)])
# Update the best-so-far solution
Gb_Fit = np.min(fitness)
index = np.argmin(fitness)
Gb_Sol = X[index, :]
# A new array to store the personal best position for each crested porcupine
Xp = X.copy()
# Optimization Process of CPO
while t <= Tmax:
r2 = np.random.rand()
for i in range(Pop_size):
U1 = np.random.rand(dim) > np.random.rand()
if np.random.rand() < np.random.rand(): # Exploration phase
if np.random.rand() < np.random.rand(): # First defense mechanism
y = (X[i, :] + X[np.random.randint(Pop_size), :]) / 2
X[i, :] = X[i, :] + np.random.randn() * abs(2 * np.random.rand() * Gb_Sol - y)
else: # Second defense mechanism
y = (X[i, :] + X[np.random.randint(Pop_size), :]) / 2
X[i, :] = U1 * X[i, :] + (1 - U1) * (y + np.random.rand() * (X[np.random.randint(Pop_size), :] - X[np.random.randint(Pop_size), :]))
else:
Yt = 2 * np.random.rand() * (1 - t / Tmax) ** (t / Tmax)
U2 = np.random.rand(dim) < 0.5 * 2 - 1
S = np.random.rand() * U2
if np.random.rand() < Tf: # Third defense mechanism
St = np.exp(fitness[i] / (np.sum(fitness) + 1e-10))
S = S * Yt * St
X[i, :] = (1 - U1) * X[i, :] + U1 * (X[np.random.randint(Pop_size), :] + St * (X[np.random.randint(Pop_size), :] - X[np.random.randint(Pop_size), :]) - S)
else: # Fourth defense mechanism
Mt = np.exp(fitness[i] / (np.sum(fitness) + 1e-10))
vt = X[i, :]
Vtp = X[np.random.randint(Pop_size), :]
Ft = np.random.rand(dim) * (Mt * (-vt + Vtp))
S = S * Yt * Ft
X[i, :] = (Gb_Sol + (alpha * (1 - r2) + r2) * (U2 * Gb_Sol - X[i, :])) - S
# Return the search agents that exceed the search space's bounds
for j in range(dim):
if X[i, j] > ub[j]:
X[i, j] = lb[j] + np.random.rand() * (ub[j] - lb[j])
elif X[i, j] < lb[j]:
X[i, j] = lb[j] + np.random.rand() * (ub[j] - lb[j])
# Calculate the fitness value of the newly generated solution
nF = fobj(X[i, :])
# Update Global & Personal best solution
if fitness[i] < nF:
X[i, :] = Xp[i, :] # Update local best solution
else:
Xp[i, :] = X[i, :]
fitness[i] = nF
if fitness[i] <= Gb_Fit:
Gb_Sol = X[i, :] # Update global best solution
Gb_Fit = fitness[i]
# Update population size
Pop_size = int(N_min + (N - N_min) * (1 - (t % (Tmax // T)) / (Tmax // T)))
t += 1 # Move to the next generation
if t > Tmax:
break
Conv_curve[t] = Gb_Fit
return Gb_Fit, Gb_Sol, Conv_curve
def initialization(SearchAgents_no, dim, ub, lb):
Boundary_no = len(ub)
if Boundary_no == 1:
Positions = np.random.rand(SearchAgents_no, dim) * (ub - lb) + lb
else:
Positions = np.zeros((SearchAgents_no, dim))
for i in range(dim):
ub_i = ub[i]
lb_i = lb[i]
Positions[:, i] = np.random.rand(SearchAgents_no) * (ub_i - lb_i) + lb_i
return Positions
```
### 说明
1. **初始化部分**:`initialization`函数用于生成初始种群位置。
2. **主循环**:在`CPO`函数中,通过嵌套的`while`和`for`循环实现优化过程。
3. **边界检查**:在更新解时,检查并修正超出搜索空间范围的值。
4. **适应度评估**:计算每个个体的适应度值,并更新全局和个人最优解。
### 注意事项
- `fobj`是目标函数,需要用户提供具体的实现。
- 数组操作使用了NumPy库,确保安装了NumPy库 (`pip install numpy`)。
- MATLAB中的某些随机数生成方式在Python中可能略有不同,但总体逻辑保持一致。
阅读全文
相关推荐
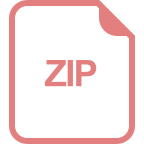
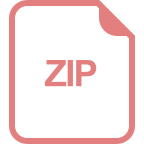
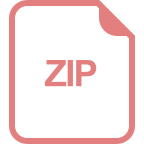
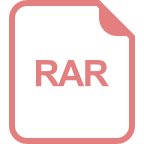
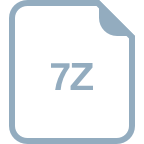
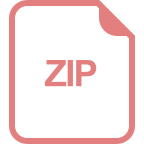
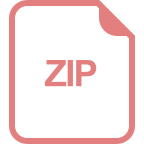
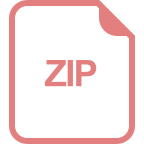
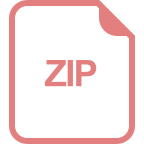
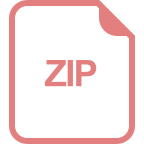
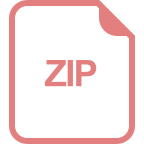
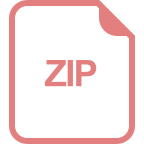
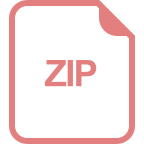
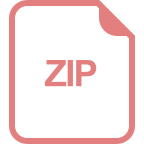
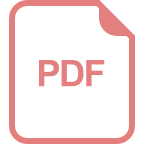
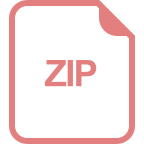
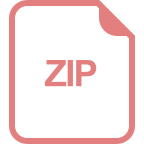
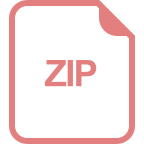