编写程序,定义有理数类,并且创建两个实例,完成有理数的相加、相乘。(相減、相除选做)
时间: 2024-11-03 07:22:41 浏览: 17
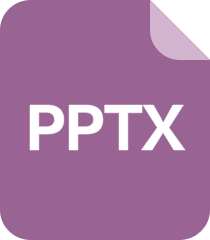
七年级数学上有理数的混合运算华东师大PPT课件.pptx
在Python中,我们可以创建一个名为`RationalNumber`的类来定义有理数,它包含分子(numerator)和分母(denominator)。以下是基本的实现,包括相加和相乘的功能,以及一个可选的相减功能,相除由于涉及到除法运算需要额外考虑除数为零的情况。
```python
class RationalNumber:
def __init__(self, numerator, denominator):
self.numerator = numerator
self.denominator = abs(denominator)
def __str__(self):
return f"{self.numerator}/{self.denominator}"
def reduce(self):
gcd = self.gcd(self.numerator, self.denominator)
self.numerator //= gcd
self.denominator //= gcd
def gcd(self, a, b):
while b != 0:
a, b = b, a % b
return a
def add(self, other):
common_denom = self.denominator * other.denominator // self.gcd(self.denominator, other.denominator)
new_numerator = (self.numerator * common_denom) + (other.numerator * self.denominator)
result = RationalNumber(new_numerator, common_denom)
result.reduce()
return result
def multiply(self, other):
numerator = self.numerator * other.numerator
denominator = self.denominator * other.denominator
result = RationalNumber(numerator, denominator)
result.reduce()
return result
def subtract(self, other):
# 如果减数的分母比被减数大,交换它们
if other.denominator > self.denominator:
other, self = self, other
# 同样计算最小公倍数
lcm = self.denominator * other.denominator // self.gcd(self.denominator, other.denominator)
# 计算差值
new_numerator = (self.numerator * lcm) - (other.numerator * self.denominator)
result = RationalNumber(new_numerator, lcm)
result.reduce()
return result
# 创建两个实例
r1 = RationalNumber(4, 2)
r2 = RationalNumber(2, 3)
# 相加
print("Addition:", r1.add(r2))
# 相乘
print("Multiplication:", r1.multiply(r2))
# 选择性相减
# print("Subtraction:", r1.subtract(r2))
# 注意:这里为了简洁,我没有显示相减的操作,因为它会比较复杂并可能导致除数为零的问题
```
上述代码实现了有理数的加法和乘法操作,并提供了一个简化版本的相减方法。如果你想要完整的相减功能,那么需要处理除数为零的情况,这通常涉及判断两个分数是否可以合并(即分母是否相同),或者转换成等价分数(例如,将其中一个转换为公共分母的分数形式)。
阅读全文
相关推荐
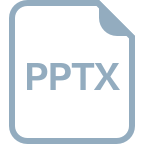
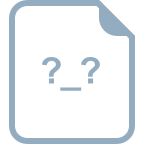

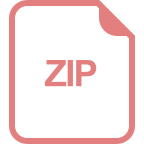
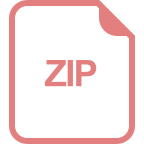
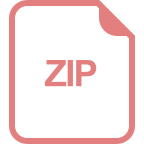
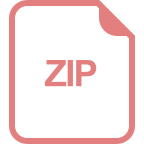
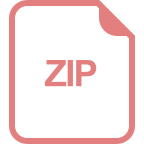
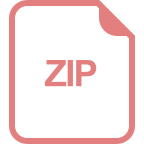
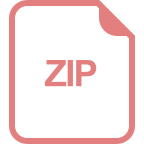
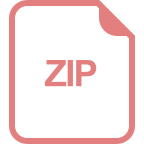
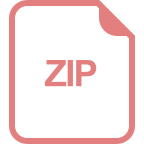
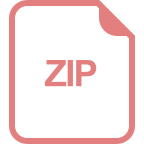
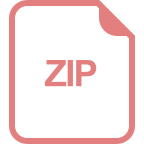