vb.net 十六进制字符串加密:获取一个 0-19 的随机数 addnum ,每一个字符的 Ascii 码增加 addnum,使得新的字符串为 0-9,A-Z 的组合。 解密:对新的字符串解密还原
时间: 2024-10-21 07:06:52 浏览: 34
在VB.NET中,你可以通过以下步骤来实现十六进制字符串的加密和解密:
**加密过程:**
1. 首先,你需要生成一个0到19之间的随机数 `addnum` 作为偏移量。
```vb
Dim randomNum As Integer = New Random().Next(0, 20)
```
2. 接下来,遍历输入字符串的每个字符,将其ASCII码值加上 `addnum`,然后转换成字符范围内的对应字母(0-9, A-Z)。如果超过了这个范围,就取模( `%` 操作符)以保持十六进制的字符集。
```vb
Dim inputString As String = "YourInputString"
Dim encryptedString As StringBuilder()
For Each c As Char In inputString
Dim newAscii As Integer = Asc(c) + randomNum Mod 65 ' ASCII of 'A' is 65
If newAscii < 48 Or newAscii > 57 AndAlso newAscii < 97 Or newAscii > 122 Then
newAscii += 26 ' Add the difference between 'A' and 'a'
End If
encryptedString.Add(Char(newAscii))
Next
encryptedString = encryptedString.ToString()
```
**解密过程:**
为了解密,你需要做相反的操作,从加密后的字符串中减去相同的偏移量 `addnum`,并确保结果仍落在正确的字符范围内。
```vb
' 解密函数
Function Decrypt(ByVal encryptedChars As String, ByVal addnum As Integer) As String
Dim decryptedString As StringBuilder()
For Each c As Char In encryptedChars
Dim asciiValue As Integer = Asc(c) - addnum Mod 65
If asciiValue < 48 Or asciiValue > 57 Then
asciiValue -= 26 ' Remove the added difference
End If
decryptedString.Add(Char(asciiValue))
Next
Return decryptedString.ToString()
End Function
' 使用解密函数
Dim decryptedString As String = Decrypt(encryptedString, randomNum)
```
现在你可以使用 `Decrypt` 函数解密得到原始的十六进制字符串。
阅读全文
相关推荐









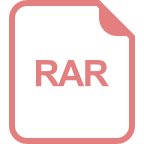

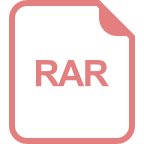




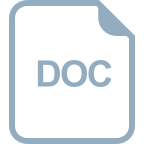
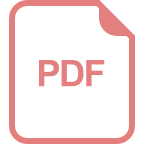