netty 搭建 websocket 服务端,客户端通过公钥方式来访问http 进行注册,注册成功后获取认证信息,并通过认证信息连接websocket端
时间: 2024-04-10 22:33:09 浏览: 203
你可以使用Netty来搭建WebSocket服务端,并通过公钥方式进行HTTP注册。以下是一个基本的示例代码:
```java
public class WebSocketServer {
public void start() {
EventLoopGroup bossGroup = new NioEventLoopGroup();
EventLoopGroup workerGroup = new NioEventLoopGroup();
try {
ServerBootstrap bootstrap = new ServerBootstrap();
bootstrap.group(bossGroup, workerGroup)
.channel(NioServerSocketChannel.class)
.childHandler(new WebSocketServerInitializer());
ChannelFuture future = bootstrap.bind(8080).sync();
future.channel().closeFuture().sync();
} catch (InterruptedException e) {
e.printStackTrace();
} finally {
bossGroup.shutdownGracefully();
workerGroup.shutdownGracefully();
}
}
public static void main(String[] args) {
WebSocketServer server = new WebSocketServer();
server.start();
}
}
```
然后,你可以创建一个 `WebSocketServerInitializer` 类来处理WebSocket连接和HTTP请求:
```java
public class WebSocketServerInitializer extends ChannelInitializer<SocketChannel> {
@Override
protected void initChannel(SocketChannel ch) throws Exception {
ChannelPipeline pipeline = ch.pipeline();
// 添加HTTP请求处理器
pipeline.addLast(new HttpServerCodec());
pipeline.addLast(new HttpObjectAggregator(65536));
pipeline.addLast(new ChunkedWriteHandler());
pipeline.addLast(new HttpHandler());
// 添加WebSocket处理器
pipeline.addLast(new WebSocketServerProtocolHandler("/websocket"));
pipeline.addLast(new WebSocketHandler());
}
}
```
在 `HttpHandler` 类中,你可以进行公钥验证和注册的逻辑处理:
```java
public class HttpHandler extends SimpleChannelInboundHandler<FullHttpRequest> {
@Override
protected void channelRead0(ChannelHandlerContext ctx, FullHttpRequest request) throws Exception {
// 进行公钥验证和注册的逻辑处理
// ...
// 注册成功后返回认证信息
FullHttpResponse response = new DefaultFullHttpResponse(HttpVersion.HTTP_1_1, HttpResponseStatus.OK);
response.content().writeBytes("Authentication successful!".getBytes());
response.headers().set(HttpHeaderNames.CONTENT_TYPE, "text/plain");
ctx.writeAndFlush(response);
ctx.channel().close();
}
}
```
最后,在 `WebSocketHandler` 类中,你可以处理WebSocket连接和消息的逻辑:
```java
public class WebSocketHandler extends SimpleChannelInboundHandler<WebSocketFrame> {
@Override
protected void channelRead0(ChannelHandlerContext ctx, WebSocketFrame frame) throws Exception {
// 处理WebSocket消息
// ...
}
@Override
public void channelActive(ChannelHandlerContext ctx) throws Exception {
// WebSocket连接建立时的处理
// ...
}
@Override
public void channelInactive(ChannelHandlerContext ctx) throws Exception {
// WebSocket连接关闭时的处理
// ...
}
}
```
这是一个简单的示例,你可以根据你的需求进行更详细的实现。希望对你有帮助!
阅读全文
相关推荐
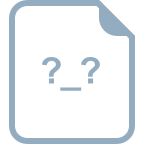
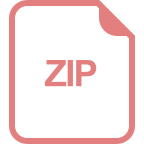
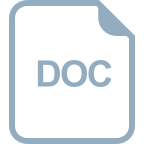
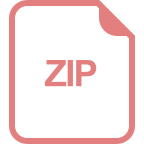
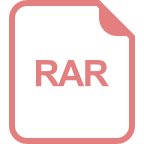
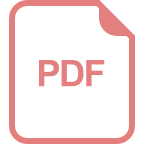
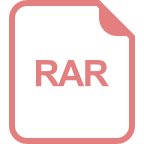
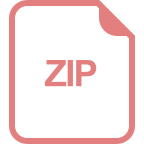
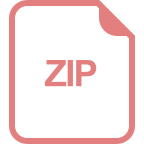
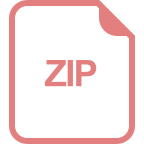
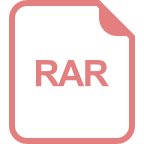
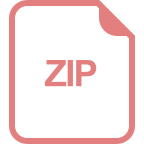
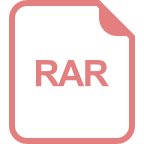
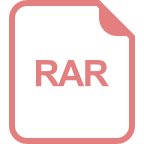
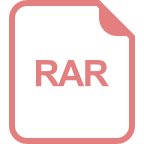
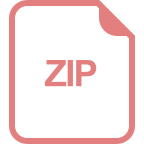
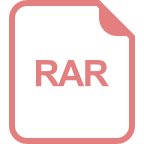
