apache的 ZipEntry压缩时中文文件名乱码解决办法
时间: 2024-12-10 18:33:25 浏览: 64
Apache的`ZipEntry`在处理包含中文文件名时可能会遇到编码问题,因为默认的编码设置可能无法正确处理非ASCII字符。为了解决这个问题,你可以按照以下步骤操作:
1. **明确文件名编码**:首先确认源文件的编码,通常是UTF-8。如果源文件不是UTF-8,需要将其转换。
2. **创建ZipEntry**时指定编码:在创建`ZipEntry`时,传入正确的编码,例如`Charset.forName("UTF-8")`。例如:
```java
ZipOutputStream zos = new ZipOutputStream(new FileOutputStream("output.zip"));
ZipEntry entry = new ZipEntry("文件名.txt");
entry.setEncoding(Charset.forName("UTF-8").name()); // 设置UTF-8编码
```
3. **使用BOM(Byte Order Mark)**:如果你的文件头包含了BOM(如UTF-8 BOM),可以考虑在文件开头添加它,以便工具能够识别编码。
4. **处理异常**:当处理可能出现编码错误的情况时,使用`try-catch`块捕获并处理`UnsupportedEncodingException`。
5. **验证压缩后的文件**:压缩后,使用支持UTF-8的工具查看压缩包内的文件名是否正确。
相关问题
java zip 中文文件名乱码_java使用zip压缩中文文件名乱码的解决办法
在Java中使用Zip压缩中文文件名可能会出现乱码的问题,这是因为Zip文件格式默认使用的是ASCII编码,而中文字符需要使用其他编码方式进行处理。解决这个问题的方法有两种:
1. 使用Apache Commons Compress库
可以使用Apache Commons Compress库来压缩文件,这个库支持多种编码方式,可以解决中文文件名乱码的问题。示例代码如下:
```java
import org.apache.commons.compress.archivers.ArchiveEntry;
import org.apache.commons.compress.archivers.zip.ZipArchiveOutputStream;
import org.apache.commons.compress.utils.IOUtils;
import java.io.*;
public class ZipUtil {
public static void zip(String sourcePath, String destPath) throws IOException {
FileOutputStream fos = new FileOutputStream(destPath);
ZipArchiveOutputStream zos = new ZipArchiveOutputStream(fos);
File file = new File(sourcePath);
String basePath = file.getParent();
compress(file, zos, basePath);
zos.close();
fos.close();
}
private static void compress(File file, ZipArchiveOutputStream zos, String basePath) throws IOException {
if (file.isDirectory()) {
File[] files = file.listFiles();
if (files.length == 0) {
String path = file.getPath().substring(basePath.length() + 1) + "/";
ArchiveEntry entry = zos.createArchiveEntry(new File(file.getPath()), path);
zos.putArchiveEntry(entry);
zos.closeArchiveEntry();
} else {
for (File f : files) {
compress(f, zos, basePath);
}
}
} else {
String path = file.getPath().substring(basePath.length() + 1);
ArchiveEntry entry = zos.createArchiveEntry(new File(file.getPath()), path);
zos.putArchiveEntry(entry);
InputStream is = new FileInputStream(file);
IOUtils.copy(is, zos);
is.close();
zos.closeArchiveEntry();
}
}
}
```
2. 使用Java的ZipOutputStream类
Java中自带的ZipOutputStream类也可以用于压缩文件,但是需要手动设置编码方式,示例代码如下:
```java
import java.io.*;
import java.nio.charset.Charset;
import java.util.zip.ZipEntry;
import java.util.zip.ZipOutputStream;
public class ZipUtil {
private static final int BUFFER_SIZE = 2 * 1024;
public static void zip(String sourcePath, String destPath) throws IOException {
FileOutputStream fos = new FileOutputStream(destPath);
ZipOutputStream zos = new ZipOutputStream(fos, Charset.forName("GBK"));
File file = new File(sourcePath);
String basePath = file.getParent();
compress(file, zos, basePath);
zos.close();
fos.close();
}
private static void compress(File file, ZipOutputStream zos, String basePath) throws IOException {
if (file.isDirectory()) {
File[] files = file.listFiles();
if (files.length == 0) {
String path = file.getPath().substring(basePath.length() + 1) + "/";
ZipEntry entry = new ZipEntry(path);
zos.putNextEntry(entry);
zos.closeEntry();
} else {
for (File f : files) {
compress(f, zos, basePath);
}
}
} else {
String path = file.getPath().substring(basePath.length() + 1);
ZipEntry entry = new ZipEntry(path);
zos.putNextEntry(entry);
InputStream is = new FileInputStream(file);
byte[] buffer = new byte[BUFFER_SIZE];
int len;
while ((len = is.read(buffer)) != -1) {
zos.write(buffer, 0, len);
}
is.close();
zos.closeEntry();
}
}
}
```
以上两种方法都可以解决中文文件名乱码的问题,具体使用哪一种方法可以根据实际需要选择。
如何在Java中利用ant.jar库来解压具有复杂多层目录结构的ZIP文件,并确保中文文件名正确处理?
为了解决这个问题,首先需要了解如何使用Apache Ant工具包中的`org.apache.tools.zip.ZipFile`类来操作ZIP文件。该类专门设计来处理包括多层目录结构和非ASCII文件名的复杂ZIP文件。在这里,我们将重点介绍如何在Java项目中实现这一功能,并且特别关注中文文件名的正确编码处理。
参考资源链接:[Java使用ant.jar解压缩多层ZIP文件夹](https://wenku.csdn.net/doc/82ehrw4jf5?spm=1055.2569.3001.10343)
在使用`org.apache.tools.zip.ZipFile`类之前,需要确保已经将ant.jar添加到了项目的依赖中。然后,可以通过以下步骤来实现解压功能:
1. 创建一个`ZipFile`实例,传入ZIP文件的路径。
2. 调用`getEntries()`方法获取ZIP文件中的所有条目(`ZipEntry`)。
3. 遍历每个`ZipEntry`,判断条目的类型(文件或目录)。
4. 如果是目录,则创建对应的目录结构。
5. 如果是文件,则创建对应的文件,并使用`ZipFile`提供的`getInputStream(ZipEntry entry)`方法读取条目的输入流,然后将数据写入到目标文件中。
在处理中文文件名时,`ZipFile`类提供了很好的编码支持,可以确保文件名在解压时不会出现乱码。这是因为`ZipFile`类在处理文件名时会正确处理Unicode字符,从而保持中文文件名的原始形态。
代码示例:
```java
ZipFile zipFile = new ZipFile(zipFilePath);
Enumeration<? extends ZipEntry> entries = zipFile.getEntries();
while (entries.hasMoreElements()) {
ZipEntry entry = entries.nextElement();
String entryName = entry.getName();
// 处理中文文件名编码问题
String decodedEntryName = new String(entryName.getBytes(
参考资源链接:[Java使用ant.jar解压缩多层ZIP文件夹](https://wenku.csdn.net/doc/82ehrw4jf5?spm=1055.2569.3001.10343)
阅读全文
相关推荐








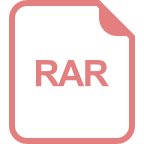






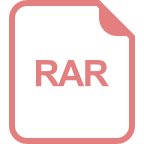
