飞机大战c语言代码加入模型部分和控制部分和显示部分
时间: 2024-10-21 10:17:59 浏览: 18
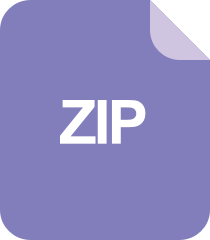
5.2 飞机大战.zip_c语言5.2_c语言小游戏_c语言飞机大战_飞机大战_飞机大战C语言

在C语言中制作一个简单的飞机大战游戏涉及到模型、控制和显示三个核心部分,通常我们会使用SDL库来创建游戏。由于C语言相对底层,这里仅给出一个简化示例,实际上你还需要考虑内存管理、数据结构和游戏逻辑优化:
**模型部分 (Model)**:
```c
typedef struct {
int x, y; // 坐标
bool is_alive; // 是否存活
} Plane;
// 初始化飞机
Plane create_plane(int x, int y) {
Plane plane = {x, y, true};
// 添加更多属性,如速度、攻击力等
return plane;
}
// 模拟飞机移动
void move_plane(Plane* plane, int dx, int dy) {
plane->x += dx;
plane->y += dy;
// 检查边界和碰撞
}
```
**控制部分 (Controller)**:
```c
void handle_input(SDL_Event& event, Plane* player) {
switch (event.type) {
case SDL_KEYDOWN:
// 使用键盘控制飞机上下左右
switch (event.key.keysym.sym) {
case SDLK_UP:
move_plane(player, 0, -1); // 上移
break;
// ...其他方向键处理
}
break;
}
}
```
**显示部分 (View or Rendering)**:
```c
void render(Plane plane, SDL_Surface* screen) {
Uint32 color = SDL_MapRGB(screen->format, 0xFF, 0x00, 0xFF); // 红色
SDL_Rect rect = {plane.x, plane.y, 30, 30}; // 飞机尺寸
SDL_FillRect(&rect, NULL, color);
SDL_BlitSurface(screen, &rect, screen, &rect);
}
void display(SDL_Surface* screen) {
// 清屏并刷新屏幕
SDL_FillRect(screen, NULL, 0);
render(*player, screen); // 画出飞机
// 显示其他游戏元素...
SDL_UpdateWindowSurface(window);
}
```
注意,这是一个非常基础的示例,实际游戏会更复杂,需要包括定时器、循环处理、碰撞检测、游戏逻辑循环等等。如果你要使用C++,可以结合上述思想,并利用现代C++特性如RAII和模板元编程来更好地组织代码。
阅读全文
相关推荐
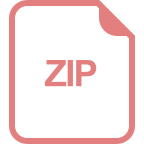
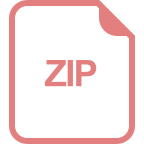
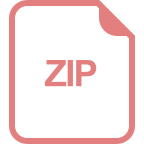
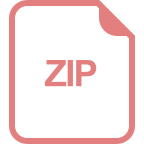
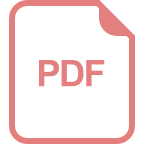
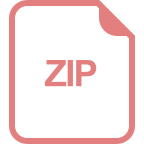
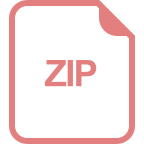
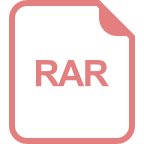
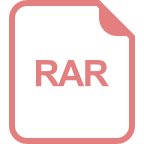
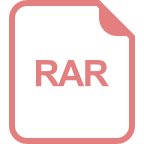
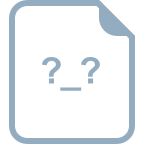