System.InvalidCastException:“Conversion from string "yyyy-mm-dd" to type 'Integer' is not valid.”
时间: 2024-10-01 19:13:29 浏览: 78
这个 `System.InvalidCastException` 错误表明你试图将字符串 "yyyy-mm-dd" 转换为整数类型(Integer),但这种转换在 .NET 中被认为是无效的。"yyyy-mm-dd" 是日期格式,而整数则是纯数字表示。在这种情况下,你的代码很可能在一个需要接收日期的地方,却传入了一个不符合预期的字符串格式。
例如,在VB.NET中,如果期望用户输入一个日期,并打算以某种方式基于这个日期做进一步的操作,那么应该先将该字符串解析为 `DateTime` 类型,而不是 `Integer`。如果你的代码中包含类似这样的片段:
```vb
Dim inputDateStr As String = "yyyy-mm-dd"
Dim dateValue As Integer = CInt(inputDateStr) ' 这里会抛出 InvalidCastException
```
正确的做法应该是:
```vb
Dim inputDateStr As String = "yyyy-mm-dd"
Dim dateValue As DateTime
If DateTime.TryParse(inputDateStr, dateValue) Then
' 现在dateValue是一个有效的DateTime对象
Else
' 输入的字符串无法转换为日期
End If
```
相关问题
System.InvalidCastException:“The SqlParameterCollection only accepts non-null SqlParameter type objects, not SqlParameter objects.”
这个异常通常是由于使用了错误的参数类型导致的。根据你提供的异常信息,看起来是在使用 `SqlParameter` 时出现了问题。
在使用 `ExecuteSqlRaw` 或 `ExecuteSqlInterpolated` 方法时,应该使用 `Microsoft.Data.SqlClient.SqlParameter` 类型而不是 `System.Data.SqlClient.SqlParameter` 类型。
请确保在代码中引用了正确的命名空间,并使用 `Microsoft.Data.SqlClient.SqlParameter` 类型来创建参数对象。
以下是一个修正该异常的示例代码:
```csharp
using Microsoft.EntityFrameworkCore;
using Microsoft.Data.SqlClient; // 确保引用了正确的命名空间
// 创建 DbContext 类
public class YourDbContext : DbContext
{
public YourDbContext(DbContextOptions<YourDbContext> options) : base(options)
{
}
// DbSet 和其他属性...
public DbSet<IPS_Invoice> IPS_Invoices { get; set; }
}
public class IPS_Invoice
{
public int IPS_ID { get; set; }
public bool BLOCK { get; set; }
public DateTime? BLOCKTIME { get; set; }
}
public class YourRepository
{
private readonly YourDbContext _dbContext;
public YourRepository(YourDbContext dbContext)
{
_dbContext = dbContext;
}
public string UpdateBlockTime(IPS_Invoice model)
{
string message = "";
try
{
int affectedRows = _dbContext.Database.ExecuteSqlInterpolated($"UPDATE IPS_Invoices SET BLOCK = true, BLOCKTIME = {DateTime.Now} WHERE IPS_ID = {model.IPS_ID}");
if (affectedRows > 0)
{
message = "True";
}
else
{
message = "No records updated";
}
}
catch (Exception e)
{
message = "False";
// 处理异常...
}
return message;
}
}
```
请确保在代码中引用了正确的 `Microsoft.Data.SqlClient` 命名空间,并使用 `Microsoft.Data.SqlClient.SqlParameter` 类型来创建参数对象。这样应该可以解决该异常。
如果问题仍然存在,请提供更多相关的代码和异常堆栈信息,以便我能够更好地帮助你。
System.InvalidCastException:“Unable to cast object of type 'System.Int64' to type 'System.Int32'.”
这个错误通常出现在将一个 Int64 类型的变量强制转换为 Int32 类型时。Int64 是 64 位整数类型,而 Int32 是 32 位整数类型,它们之间不能直接转换。如果你需要将 Int64 类型的变量转换为 Int32 类型,可以使用 Convert.ToInt32 方法进行转换,或者你可以先将 Int64 类型的变量转换为字符串,然后再将字符串转换为 Int32 类型。例如:
```csharp
long myLong = 1234567890;
int myInt = Convert.ToInt32(myLong); // 使用 Convert.ToInt32 进行转换
// 或者
string myString = myLong.ToString();
myInt = int.Parse(myString); // 先将 long 类型的变量转换为字符串,再将字符串转换为 int 类型
```
在进行强制类型转换时,一定要确保转换的类型是兼容的,否则就会抛出类似的异常。
相关推荐
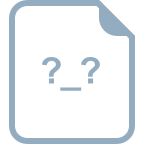
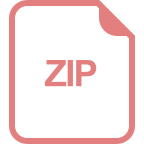
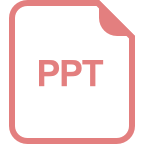












