visual studio如何编写 C# 接口网关
时间: 2024-10-12 22:12:17 浏览: 54
在Visual Studio中编写C#接口网关,通常涉及到以下几个步骤:
1. **项目创建**:首先,打开Visual Studio,新建一个ASP.NET Core Web Application项目,选择"API"模板,因为API Gateway通常是基于HTTP的RESTful服务。
2. **添加依赖项**:在项目文件中,添加`Microsoft.AspNetCore.Mvc.NewtonsoftJson`和`Microsoft.Azure.Functions.Worker`等库,如果计划使用Azure Functions作为后端处理逻辑。
3. **设计接口**:使用`Microsoft.AspNetCore.Http.Abstractions`包创建接口类,定义API的路由、方法、参数类型等。例如,你可以创建一个`IGatewayService`接口,用于定义基本的请求处理逻辑。
```csharp
public interface IGatewayService
{
[HttpGet]
Task<string> GetResources([FromQuery] string param);
}
```
4. **实现接口**:为接口创建具体的实现类,如`GatewayController`,并在其中注入所需的服务和中间件。
```csharp
public class GatewayController : ControllerBase, IGatewayService
{
private readonly YourDependency _dependency;
public GatewayController(YourDependency dependency)
{
_dependency = dependency;
}
[HttpGet]
public async Task<ActionResult<string>> GetResources(string param)
{
// 实现业务逻辑,例如路由到其他微服务或直接返回结果
var result = await _dependency.ProcessRequest(param);
return Ok(result);
}
}
```
5. **路由配置**:在Startup.cs中配置IEndpointRouteBuilder,将接口映射到实际的URL路径上。
```csharp
app.UseEndpoints(endpoints =>
{
endpoints.MapControllers().RequireAuthorization();
});
```
6. **部署**:如果是在Azure中,可以选择部署到Web App或Azure Functions,根据需求配置相应的设置。
阅读全文
相关推荐
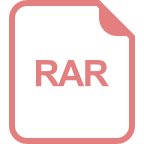
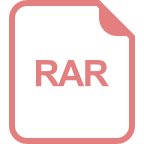
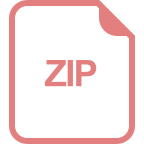
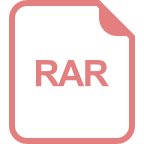
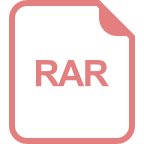
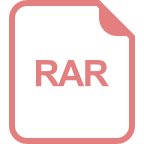
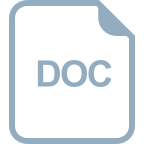
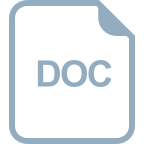
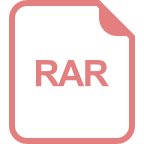
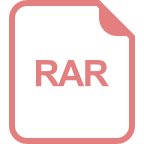
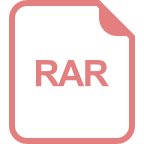
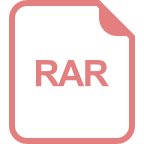
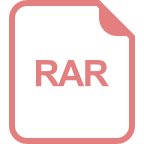
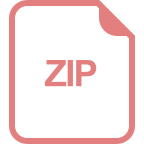
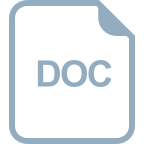
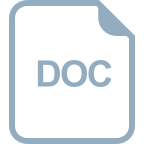
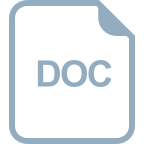
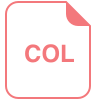
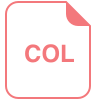