java 工具类代码
时间: 2025-01-09 22:44:32 浏览: 1
### Java 工具类代码示例
工具类通常用于封装一组静态方法,这些方法提供特定功能而不必创建对象实例。下面是一些常见的 Java 工具类及其代码实现。
#### 字符串处理工具类 `StringUtils`
此工具类提供了几个常用的字符串操作函数:
```java
public final class StringUtils {
private StringUtils() {
throw new UnsupportedOperationException("This is a utility class and cannot be instantiated");
}
public static boolean isEmpty(String str) {
return str == null || str.trim().isEmpty();
}
public static String reverseString(String input) {
if (input == null) {
return null;
}
return new StringBuilder(input).reverse().toString();
}
public static int countOccurrences(String str, char ch) {
if (str == null) {
return 0;
}
int count = 0;
for (int i = 0; i < str.length(); i++) {
if (str.charAt(i) == ch) {
++count;
}
}
return count;
}
}
```
该工具类定义了一个私有构造器防止被实例化,并实现了三个常用的方法:判断字符串是否为空、反转字符串以及统计字符出现次数[^5]。
#### 文件读取工具类 `FileUtils`
文件操作也是编程中经常遇到的任务之一。这里展示如何编写一个简单的文件读写工具类:
```java
import java.io.*;
import java.nio.file.Files;
import java.util.List;
public final class FileUtils {
private FileUtils() {}
public static List<String> readFileLines(String filePath) throws IOException {
return Files.readAllLines(Paths.get(filePath));
}
public static void writeFileContent(String content, String filePath) throws IOException {
try (BufferedWriter writer = new BufferedWriter(new FileWriter(filePath))) {
writer.write(content);
}
}
}
```
这段代码展示了两个基本的功能——从给定路径加载整个文件到内存列表里;向指定位置保存一段文本内容。
阅读全文
相关推荐



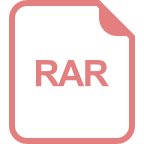

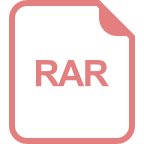

