实现“.”一个链式调用的类
时间: 2024-10-24 17:10:29 浏览: 35
链式调用是一种常见的设计模式,在JavaScript中特别常见,尤其是在处理事件处理器或操作数组等场景。它通常涉及到创建一个类,这个类包含一系列方法,每个方法返回对自身的引用,以便用户可以连续地调用它们,形成类似"."操作的链。
举个例子,假设我们有一个`ChainableOperation`类:
```java
class ChainableOperation {
private Operation current;
public ChainableOperation(Operation operation) {
this.current = operation;
}
public ChainableOperation then(Operation nextOperation) {
this.current = new OperationChaining(this.current, nextOperation);
return this; // 返回自身实例,允许链式调用
}
public void execute() {
current.execute();
}
}
class Operation {
// 基础操作实现
abstract void execute();
}
class OperationChaining extends Operation {
private final Operation first;
private final Operation second;
public OperationChaining(Operation first, Operation second) {
this.first = first;
this.second = second;
}
@Override
void execute() {
first.execute();
second.execute();
}
}
```
在这个例子中,`ChainableOperation`类允许你在`then()`方法中添加更多的操作,并通过`execute()`触发整个链。用户可以像下面这样使用:
```java
ChainableOperation op1 = new ChainableOperation(new Add(5));
ChainableOperation op2 = op1.then(new Multiply(2));
op2.execute(); // 先加5,再乘以2
```
阅读全文
相关推荐

















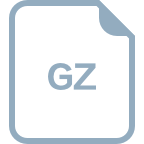
