单链表把任意给定的两个一元多项式P(x),Q(x)输入计算机,计算它们和并输出计算结果的c++代码
时间: 2024-10-17 21:11:26 浏览: 13
在C++中,单链表通常用于存储数值元素,如果要用链表表示一元多项式,可以将系数和指数作为节点的数据成员。然而,直接在链表上做多项式的加法操作并不直观,因为链表不适合直接进行多项式运算。通常我们会先将其转换为数组或者动态数据结构(如树)来方便处理。
这里是一个简单的例子,展示如何用C++表示一元多项式,并提供一个将两个多项式相加的基本思路,假设我们有一个`PolynomialNode`类来存储节点:
```cpp
class PolynomialNode {
public:
int coefficient;
int exponent;
PolynomialNode(int c = 0, int e = 0) : coefficient(c), exponent(e) {}
};
// 使用双向链表来存储多项式
struct PolynomialList {
private:
PolynomialNode* head;
PolynomialNode* tail;
public:
void addTerm(int c, int e) {
PolynomialNode* newNode = new PolynomialNode(c, e);
if (!head) {
head = tail = newNode;
} else {
tail->exponent == 0 ? tail->coefficient += c : tail->next = newNode; // 如果尾节点不是最高次项,则直接累加
tail = newNode; // 更新尾节点
}
}
void print() {
PolynomialNode* current = head;
while (current) {
std::cout << current->coefficient << "x^" << current->exponent << " + ";
current = current->next;
}
std::cout << "0\n"; // 输出终止0
}
PolynomialList operator+(const PolynomialList& other) {
PolynomialList result;
PolynomialNode* current1 = head, *current2 = other.head;
PolynomialNode* temp = nullptr;
while (current1 && current2) {
int sum = current1->coefficient + current2->coefficient;
result.addTerm(sum, std::max(current1->exponent, current2->exponent));
current1 = current1->next;
current2 = current2->next;
}
// 添加剩余的项(如果有的话)
while (current1) {
result.addTerm(current1->coefficient, current1->exponent);
current1 = current1->next;
}
while (current2) {
result.addTerm(current2->coefficient, current2->exponent);
current2 = current2->next;
}
return result;
}
};
int main() {
PolynomialList p1, p2;
// 添加一元多项式的项到p1和p2...
PolynomialList result = p1 + p2;
result.print(); // 输出结果
return 0;
}
```
这个代码仅作演示,实际应用中可能需要优化,比如考虑合并相同次数的项。注意这并不是真正意义上的“链表”,因为链表通常是递归数据结构,而此处使用了更为紧凑的顺序存储形式。
阅读全文
相关推荐
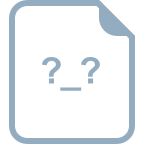
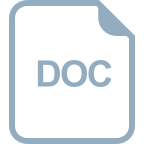
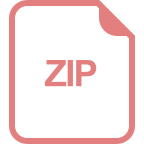
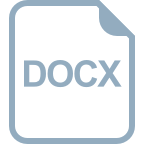
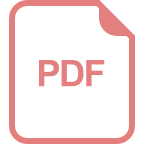
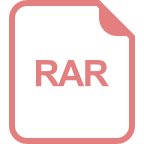
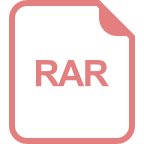
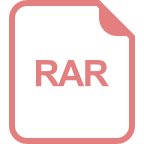
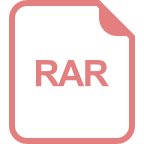
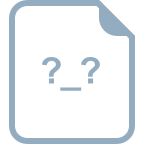
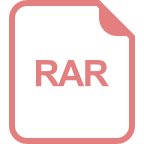
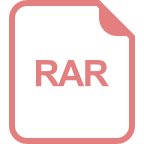
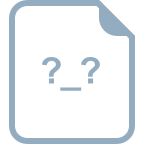
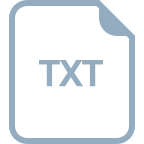
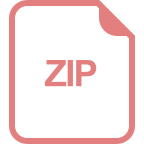