redux和reducer
时间: 2024-06-13 22:08:04 浏览: 242
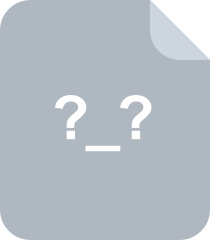
redux_reducer
Redux是一个用于JavaScript应用程序的可预测状态容器,它可以帮助我们更好地管理应用程序的状态。而Reducer是Redux中用于处理状态变化的函数,它接收先前的状态和一个动作对象作为参数,并返回新的状态。Reducer的设计灵感来自于JavaScript中的Array.prototype.reduce()方法,因为它们都是将先前的值和当前的值组合成一个新值的过程。在Redux中,Reducer通常是纯函数,它们不会修改先前的状态,而是返回一个新的状态对象。
下面是一个简单的Redux应用程序,其中包含一个Reducer:
```javascript
// 定义初始状态
const initialState = {
count: 0
};
// 定义Reducer
function counterReducer(state = initialState, action) {
switch (action.type) {
case 'INCREMENT':
return { count: state.count + 1 };
case 'DECREMENT':
return { count: state.count - 1 };
default:
return state;
}
}
// 创建Redux store
const { createStore } = Redux;
const store = createStore(counterReducer);
// 订阅状态变化
store.subscribe(() => {
console.log(store.getState());
});
// 分发动作
store.dispatch({ type: 'INCREMENT' }); // 输出:{ count: 1 }
store.dispatch({ type: 'INCREMENT' }); // 输出:{ count: 2 }
store.dispatch({ type: 'DECREMENT' }); // 输出:{ count: 1 }
```
在上面的例子中,我们定义了一个名为`counterReducer`的Reducer函数,它接收先前的状态和一个动作对象作为参数,并返回一个新的状态对象。我们还创建了一个Redux store,并将`counterReducer`作为参数传递给`createStore`函数。最后,我们分发了一些动作,并在控制台中打印了状态变化。
阅读全文
相关推荐
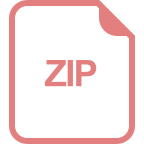
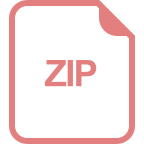
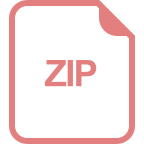
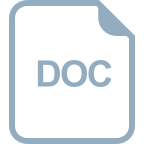

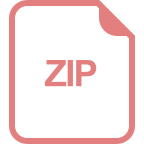
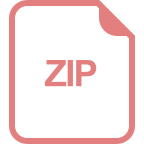
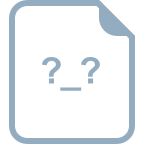
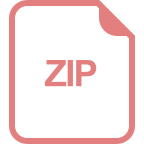
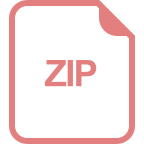
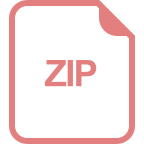
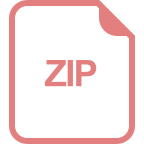
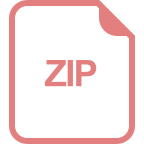
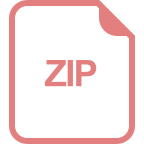
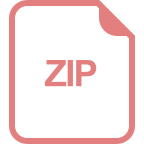
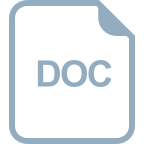