某软件公司有n名员工,每名员工有姓名、职务、工号等属性,请把所有员工建立一个线性表,建立离职、入职,查询函数,当有员工商职成入职时,修改线性表,并且根据输出格式中的要求输出。(要求线性表采用动态分配的顺序
时间: 2024-09-23 11:03:00 浏览: 44
要创建一个动态数组(线性表)来存储员工信息,并实现离职、入职操作以及查询功能,你可以使用结构体定义员工的基本属性,然后用动态内存分配创建一个动态数组。这里是一个简单的示例:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
// 定义员工结构体
typedef struct {
char name[50];
char position[50];
int id;
} Employee;
// 动态数组的大小
#define MAX_EMPLOYEES 100
// 动态创建和初始化员工数组
Employee* create_employee_array(int n) {
Employee* employees = (Employee*)malloc(n * sizeof(Employee));
if (employees == NULL) {
printf("Memory allocation failed.\n");
return NULL;
}
for (int i = 0; i < n; ++i) {
employees[i].name[0] = '\0';
employees[i].position[0] = '\0';
employees[i].id = 0;
}
return employees;
}
// 离职函数,从数组中移除指定员工
void leave(Employee** employees, int index) {
if (index >= 0 && index < MAX_EMPLOYEES) {
memmove(&employees[index], &employees[index + 1], (MAX_EMPLOYEES - index - 1) * sizeof(Employee));
free(employees[MAX_EMPLOYEES - 1]);
employees[MAX_EMPLOYEES - 1] = NULL;
} else {
printf("Invalid index.\n");
}
}
// 入职函数,添加新员工到数组尾部
void join(Employee** employees, const char* name, const char* position, int id) {
if (*employees == NULL) {
*employees = create_employee_array(1);
} else if (MAX_EMPLOYEES == sizeof(*employees) / sizeof(Employee)) {
printf("No more space in the array, consider resizing it.\n");
return;
}
(*employees)[MAX_EMPLOYEES - 1].name = strdup(name);
(*employees)[MAX_EMPLOYEES - 1].position = strdup(position);
(*employees)[MAX_EMPLOYEES - 1].id = id;
}
// 查询函数,根据条件查找并打印符合条件的员工信息
void search_and_print(Employee* employees, int n) {
for (int i = 0; i < n; ++i) {
if (strcmp(employees[i].position, "new position") == 0) { // 示例:假设我们要搜索"新职位"
printf("ID: %d, Name: %s, Position: %s\n", employees[i].id, employees[i].name, employees[i].position);
}
}
}
// 主程序入口
int main() {
int num_employees = 5; // 假设开始有5名员工
Employee* employees = create_employee_array(num_employees);
// 员工入职示例
join(&employees, "John Doe", "Developer", 1001);
// 搜索新职位的员工
search_and_print(employees, num_employees);
// 假设员工2离职
leave(&employees, 1);
// 更新后再次搜索
search_and_print(employees, num_employees);
free(employees); // 手动释放内存
return 0;
}
```
相关推荐
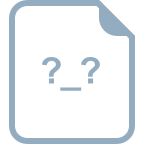
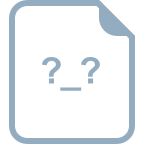
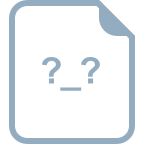

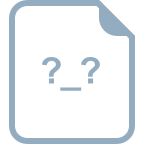
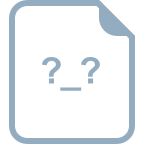
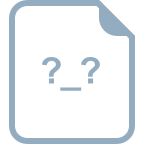
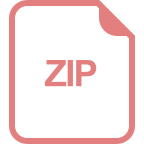
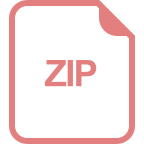
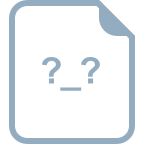
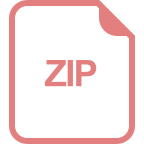
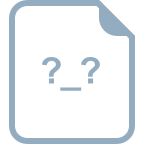
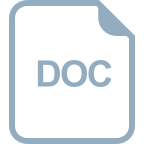
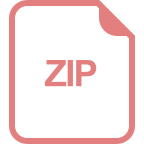
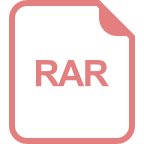
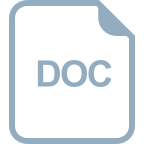
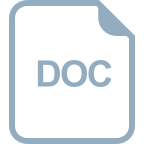
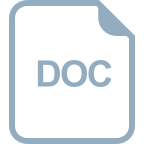