springboot test mapper注入
时间: 2023-11-15 11:48:45 浏览: 195
在Spring Boot中测试Mapper接口的注入,可以使用@SpringBootTest和@Autowired注解来实现。在测试类上添加@SpringBootTest注解,它会加载整个Spring容器,包括所有的Bean,并将它们注入到测试类中。然后使用@Autowired注解将Mapper接口注入到测试类中的相应字段中。
代码示例:
```
@SpringBootTest
public class ProductMapperTest {
@Autowired
private ProductMapper productMapper;
// 测试方法...
}
```
相关问题
springboot通过mapper连接mysql
在Spring Boot中,我们通常使用MyBatis作为ORM框架来访问数据库。MyBatis是一种基于Java的持久层框架,它可以通过XML或注解的方式来配置SQL语句和映射关系,并且提供了灵活的SQL执行和结果映射机制。
下面是通过Mapper连接MySQL的步骤:
1. 添加依赖
在pom.xml中添加以下依赖:
```
<dependency>
<groupId>org.mybatis.spring.boot</groupId>
<artifactId>mybatis-spring-boot-starter</artifactId>
<version>2.1.4</version>
</dependency>
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
<version>8.0.26</version>
</dependency>
```
2. 配置数据源
在application.properties或application.yml中配置数据源信息:
```
spring.datasource.url=jdbc:mysql://localhost:3306/test?useUnicode=true&characterEncoding=utf8&serverTimezone=UTC
spring.datasource.username=root
spring.datasource.password=123456
spring.datasource.driver-class-name=com.mysql.cj.jdbc.Driver
```
3. 配置Mapper
在Mapper接口中定义SQL语句的方法,可以使用注解或XML文件来配置SQL语句和映射关系。例如:
```
@Mapper
public interface UserMapper {
@Select("SELECT * FROM user WHERE id = #{id}")
User findById(@Param("id") Long id);
@Insert("INSERT INTO user(name, age) VALUES(#{name}, #{age})")
int insert(User user);
@Update("UPDATE user SET name=#{name}, age=#{age} WHERE id=#{id}")
int update(User user);
@Delete("DELETE FROM user WHERE id=#{id}")
int deleteById(@Param("id") Long id);
}
```
4. 添加Mapper扫描
在启动类上添加@MapperScan注解,指定Mapper接口所在的包路径,例如:
```
@SpringBootApplication
@MapperScan("com.example.demo.mapper")
public class DemoApplication {
public static void main(String[] args) {
SpringApplication.run(DemoApplication.class, args);
}
}
```
5. 使用Mapper
在需要使用Mapper的地方,通过@Autowired注解将Mapper注入进来,例如:
```
@Service
public class UserService {
@Autowired
private UserMapper userMapper;
public User findById(Long id) {
return userMapper.findById(id);
}
public int insert(User user) {
return userMapper.insert(user);
}
public int update(User user) {
return userMapper.update(user);
}
public int deleteById(Long id) {
return userMapper.deleteById(id);
}
}
```
以上就是通过Mapper连接MySQL的步骤。需要注意的是,如果使用XML文件来配置SQL语句和映射关系,需要在Mapper接口上添加@Mapper注解,并在application.properties或application.yml中添加以下配置:
```
mybatis.mapper-locations=classpath:mybatis/mapper/*.xml
```
其中,mybatis.mapper-locations配置了XML文件所在的路径。
SpringBoot测试类 mapper注入为null
如果在SpringBoot测试类中,mapper注入为null,可能是因为没有正确地配置测试环境。可以尝试以下方法:
1. 确认在测试类上方添加了注解@RunWith(SpringRunner.class)和@SpringBootTest注解,并且指定了正确的启动类。
2. 确认在测试类中注入的mapper的类型和名称与接口和实现类的定义一致,并且使用了@Autowired或@Resource注解进行注入。
3. 确认在测试类中使用的数据库配置和application.properties或yml文件中的配置一致。
4. 如果仍然无法解决问题,可以尝试使用@MapperScan注解扫描mapper接口所在的包路径。
希望这些方法可以帮助你解决问题。
阅读全文
相关推荐
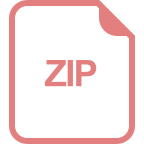
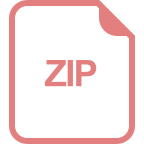








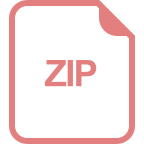
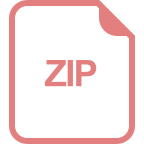
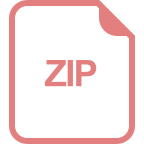
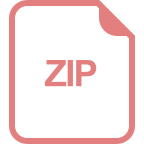
