鲸鱼优化算法-双向长短期记忆
时间: 2023-12-27 11:04:03 浏览: 182
鲸鱼优化算法(Beluga whale optimization,BWO)是一种基于仿生学的优化算法,模拟了白鲸游泳、觅食和“鲸鱼坠落”行为。而双向长短期记忆(BiLSTM)是一种常用于时间序列预测的神经网络模型。将这两者结合起来,可以使用BWO算法来优化BiLSTM的权值和阈值,从而提高时间序列预测的准确性。
以下是使用BWO算法优化BiLSTM的示例代码:
```python
# 导入必要的库
import numpy as np
from keras.models import Sequential
from keras.layers import Dense, LSTM, Bidirectional
from keras.optimizers import Adam
from bwo import BWO # 导入BWO算法实现
# 构建BiLSTM模型
model = Sequential()
model.add(Bidirectional(LSTM(64, activation='relu'), input_shape=(n_steps, n_features)))
model.add(Dense(1))
model.compile(optimizer=Adam(learning_rate=0.01), loss='mse')
# 定义适应度函数
def fitness_func(solution):
# 将解向量转换为权值和阈值
w1 = solution[:n_features * n_units].reshape((n_features, n_units))
b1 = solution[n_features * n_units:n_features * n_units + n_units]
w2 = solution[n_features * n_units + n_units:].reshape((n_units * 2, 1))
b2 = solution[-1]
# 设置权值和阈值
model.layers[0].set_weights([w1, b1, w2, b2])
# 训练模型并计算MAE
model.fit(X_train, y_train, epochs=50, verbose=0)
y_pred = model.predict(X_test)
mae = np.mean(np.abs(y_test - y_pred))
return 1 / (mae + 1e-6)
# 定义BWO算法参数
n_agents = 30
n_iterations = 100
lb = [-1] * (n_features * n_units + n_units * 2 + 1)
ub = [1] * (n_features * n_units + n_units * 2 + 1)
# 运行BWO算法
bwo = BWO(fitness_func, lb, ub, n_agents, n_iterations)
best_solution, best_fitness = bwo.run()
# 输出最优解和最优适应度
print('Best solution:', best_solution)
print('Best fitness:', best_fitness)
```
阅读全文
相关推荐
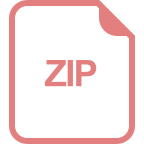
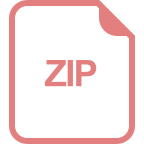
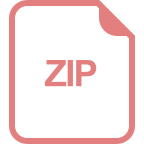
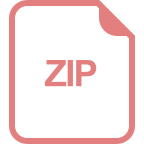
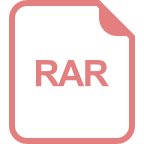
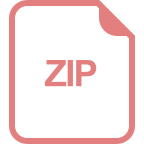
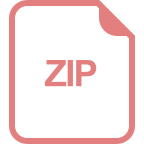
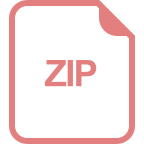
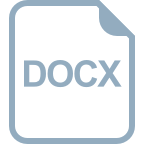
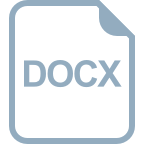
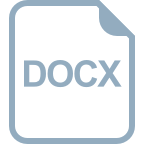
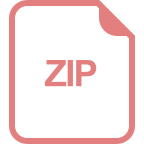
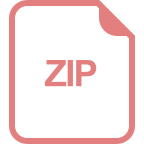
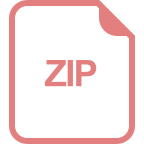
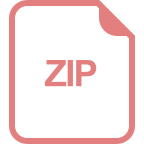
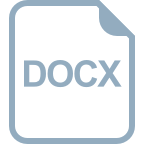

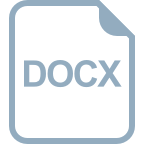
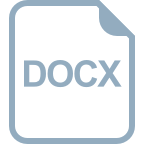