spring自定义注解
时间: 2023-08-15 07:09:42 浏览: 144
Spring框架允许我们自定义注解,用于标记特定的类、方法或属性,并通过反射来获取或处理这些注解。下面是创建自定义注解的步骤:
1. 创建一个新的Java类,用于定义自定义注解。注解需要使用`@interface`关键字进行声明。例如:
```java
package com.example.annotations;
import java.lang.annotation.ElementType;
import java.lang.annotation.Retention;
import java.lang.annotation.RetentionPolicy;
import java.lang.annotation.Target;
@Retention(RetentionPolicy.RUNTIME)
@Target(ElementType.TYPE)
public @interface CustomAnnotation {
// 在这里定义注解的属性
String value() default "";
}
```
在上面的例子中,我们创建了一个名为`CustomAnnotation`的自定义注解,该注解被定义为在运行时可见,并且可以应用于类级别。
2. 在类中使用自定义注解。例如:
```java
package com.example;
import com.example.annotations.CustomAnnotation;
@CustomAnnotation("Hello, world!")
public class MyClass {
// 类的具体实现
}
```
在上面的例子中,我们在`MyClass`类上使用了自定义注解`CustomAnnotation`,并传递了一个字符串参数作为注解的值。
3. 使用反射获取自定义注解信息。例如:
```java
package com.example;
import com.example.annotations.CustomAnnotation;
public class Main {
public static void main(String[] args) {
Class<?> clazz = MyClass.class;
CustomAnnotation annotation = clazz.getAnnotation(CustomAnnotation.class);
if (annotation != null) {
String value = annotation.value();
System.out.println("Annotation value: " + value);
}
}
}
```
在上面的例子中,我们使用反射获取`MyClass`类上的`CustomAnnotation`注解,并获取注解的值。
这就是使用Spring框架自定义注解的基本步骤。你可以根据实际需求在注解中定义不同的属性,并根据需要在应用程序中使用这些自定义注解。
阅读全文
相关推荐
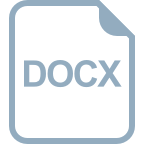
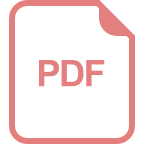
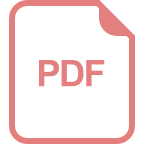
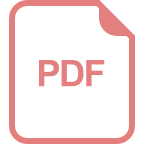
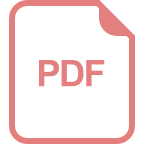
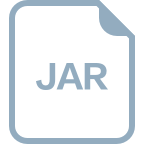
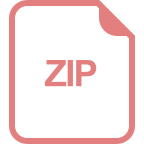
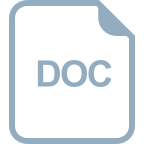


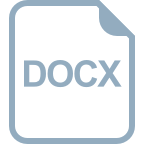
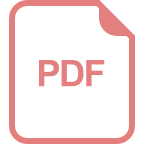
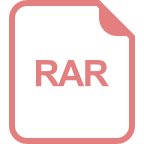
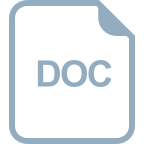

