selenium下拉框里的滚动条到某个元素出现
时间: 2024-11-03 15:21:46 浏览: 56
在Selenium中,处理网页下拉框的滚动条通常涉及到JavaScript模拟用户操作,因为浏览器的API并不直接提供这样的功能。当你需要滚动下拉列表直到特定元素可见时,可以使用`WebDriverWait`配合`ExpectedConditions`来实现。以下是一个基本步骤:
1. 首先,导入所需的模块:
```python
from selenium.webdriver.common.by import By
from selenium.webdriver.support.ui import WebDriverWait
from selenium.webdriver.support import expected_conditions as EC
```
2. 确定元素的位置,比如它的CSS选择器或XPath:
```python
element_locator = (By.CSS_SELECTOR, "select#your_dropdown") # 使用CSS选择器或其他适合的选择器
```
3. 创建一个`WebDriverWait`实例,并设置超时时间,等待元素出现或滚动条达到底部:
```python
wait = WebDriverWait(driver, 10) # 超时时间为10秒
```
4. 定义条件函数,检查元素是否出现在视窗中(这通常意味着滚动条到达了该元素):
```python
def element_visible(element):
return EC.visibility_of_element_located(element)
```
5. 实现滚动:
```python
scroll_to_bottom = False
while not wait.until(element_visible(element_locator)) and not scroll_to_bottom:
driver.execute_script("window.scrollTo(0, document.body.scrollHeight);")
scroll_to_bottom = True # 如果连续两次都没有找到元素,则假设已经滚动到底部
```
6. 最后检查元素是否已显示:
```python
if wait.until(element_visible(element_locator)):
print(f"Element {element_locator} is now visible.")
else:
print("Failed to find the element after scrolling.")
```
阅读全文
相关推荐
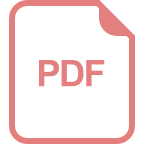
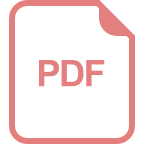
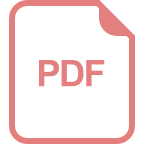















