用Cpp语言写一个算法实现统计在输入字符串中各个不同字符出现的频度。(字符串中的合法字符A~Z这26个字母和0~9这10个数字)
时间: 2024-11-03 17:23:35 浏览: 34
在C++中,我们可以使用`std::map`来实现这个功能,因为它能自动将键映射到值,并提供O(1)的时间复杂度来进行查找和插入操作。这里是一个简单的算法:
```cpp
#include <iostream>
#include <string>
#include <map>
std::map<char, int> countCharacters(const std::string& input) {
std::map<char, int> charCount;
for (char c : input) {
// 确保字符在合法范围内
if ('A' <= c && c <= 'Z' || 'a' <= c && c <= 'z' || '0' <= c && c <= '9') {
charCount[c]++;
}
}
return charCount;
}
int main() {
std::string str = "Hello World! 12345";
std::map<char, int> freq = countCharacters(str);
// 输出每个字符及其频率
for (const auto& pair : freq) {
std::cout << "Character: " << pair.first << ", Frequency: " << pair.second << '\n';
}
return 0;
}
```
在这个程序中,我们首先创建了一个空的`std::map`,然后遍历输入字符串中的每一个字符。如果字符是字母或数字,我们就将其添加到映射中并增加计数。最后,我们在主函数中打印出每个字符及其出现的次数。
阅读全文
相关推荐
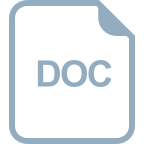
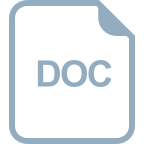
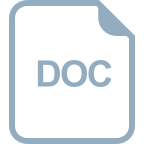



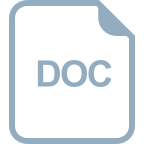
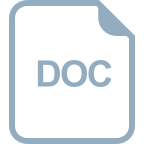
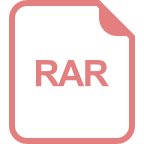
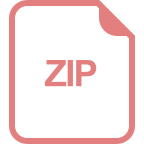
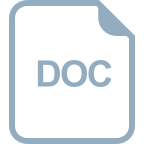
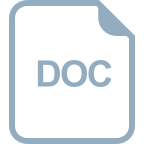
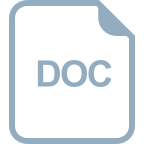
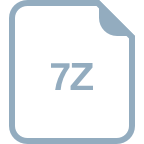
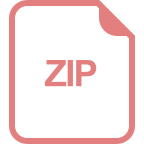
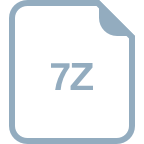