使用c++1.针对稠密矩阵和稀疏矩阵,设计实现矩阵类,要求包含矩阵访问某位置(i,j)元素的操作,以及加法、乘法、转置操作等。2.设计测试样例验证设计实现的正确性。
时间: 2024-10-23 17:06:17 浏览: 24
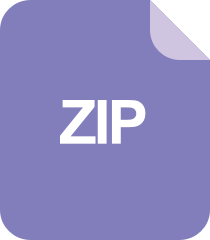
数据结构—三元组表法实现稀疏矩阵的转置.zip_WEB开发_Visual_C++_
在C++中,对于稠密矩阵(即所有元素都是连续存储的矩阵)和稀疏矩阵(只有一小部分非零元素),我们可以分别设计两个不同的矩阵类:
**稠密矩阵类(DenseMatrix)**:
```cpp
class DenseMatrix {
private:
int rows, cols;
std::vector<std::vector<int>> data;
public:
DenseMatrix(int r, int c): rows(r), cols(c) { data.resize(rows, std::vector<int>(cols, 0)); }
// 访问元素
int get(int i, int j) const { return data[i][j]; }
// 加法
void add(const DenseMatrix& other, int scalar = 1) {
if (rows != other.rows || cols != other.cols)
throw std::invalid_argument("Matrices must have the same dimensions.");
for (int i = 0; i < rows; ++i)
for (int j = 0; j < cols; ++j)
data[i][j] += other.data[i][j] * scalar;
}
// 乘法(假设是对自乘)
void multiply(DenseMatrix& result, DenseMatrix& multiplier) {
if (cols != multiplier.rows)
throw std::runtime_error("Number of columns in first matrix must be equal to number of rows in second matrix");
result = DenseMatrix(rows, multiplier.cols);
for (int i = 0; i < rows; ++i)
for (int k = 0; k < cols; ++k)
for (int j = 0; j < multiplier.cols; ++j)
result.data[i][j] += data[i][k] * multiplier.data[k][j];
}
// 转置
DenseMatrix transpose() {
DenseMatrix transposed(cols, rows);
for (int i = 0; i < rows; ++i)
for (int j = 0; j < cols; ++j)
transposed.data[j][i] = data[i][j];
return transposed;
}
};
```
**稀疏矩阵类(SparseMatrix)**:
由于稀疏矩阵大部分空间用于存储非零元素,可以采用压缩存储(如邻接列表或二叉搜索树)来节省空间。这里给出一个基本结构,实际应用中可能需要更复杂的数据结构:
```cpp
class SparseMatrix {
private:
std::vector<std::pair<int, int>> nonzeros; // 存储索引值对
int rows, cols;
public:
SparseMatrix(int r, int c): rows(r), cols(c) {}
// 省略非零元素的添加、删除、查找等操作...
int get(int i, int j) const {
for (const auto& entry : nonzeros) {
if (entry.first == i && entry.second == j)
return *entry第三个元素;
}
return 0; // 如果不存在,则返回0或其他默认值
}
// 省略加法、乘法、转置的具体实现...
};
```
**测试样例**:
```cpp
void test(DenseMatrix dense1, DenseMatrix dense2, DenseMatrix expectedAdd, int scalar = 1) {
dense1.add(dense2, scalar);
assert(dense1 == expectedAdd); // 检查加法是否正确
}
void testMultiply(DenseMatrix dense1, DenseMatrix dense2, DenseMatrix expectedProduct) {
DenseMatrix product;
dense1.multiply(product, dense2);
assert(product == expectedProduct); // 检查乘法是否正确
}
// 其他测试用例,例如转置操作
```
这些只是基本的实现和测试框架,实际应用中需要考虑更多的细节,比如异常处理、性能优化等。
阅读全文
相关推荐
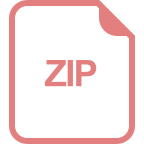
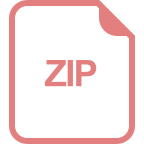
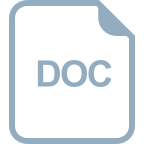
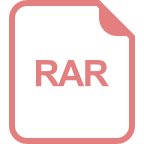
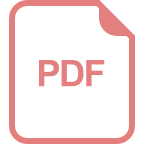
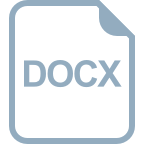
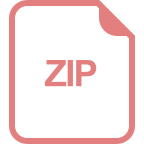
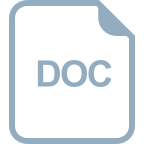
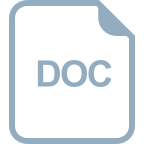
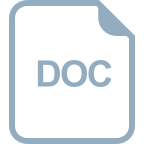
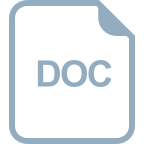
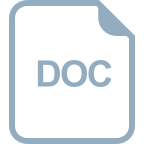
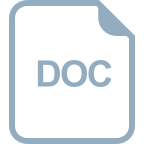
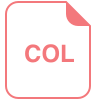
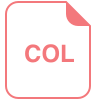


