生产者与消费者爬虫 Python 详细案例
时间: 2024-10-10 13:05:18 浏览: 33
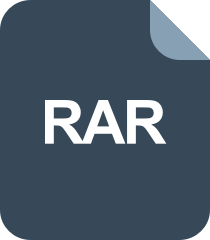
python代码爬虫案例代码

生产者消费者模型在Python中常用于编写高效的并发爬虫系统,它模拟了现实生活中生产者(如网页抓取程序)和消费者(如数据处理程序)之间的协作。这里我们可以使用内置的`queue`模块来构建这个模型。
首先,我们需要创建两个类:`Producer`(生产者)和`Consumer`(消费者)。生产者负责从网络上抓取网页,并将内容添加到队列中;消费者则从队列中取出网页内容并进行分析。
```python
import queue
import requests
class Producer:
def __init__(self, urls_queue):
self.urls_queue = urls_queue
def crawl(self):
while True:
url = self.urls_queue.get()
if url is None: # 消费者结束标志
break
response = requests.get(url)
content = response.text
self.urls_queue.task_done() # 队列任务完成
print(f"Crawled {url}, content length: {len(content)}")
class Consumer:
def __init__(self, content_queue, processor):
self.content_queue = content_queue
self.processor = processor
def process(self):
while True:
content = self.content_queue.get()
if content is None: # 生产者结束标志
break
processed_data = self.processor(content)
print(f"Processed data from {content}: {processed_data}")
self.content_queue.task_done()
# 使用示例
urls_to_crawl = ["https://example.com", "https://example.org"]
content_queue = queue.Queue()
producer = Producer(content_queue)
consumer = Consumer(content_queue, lambda x: len(x))
# 启动生产者和消费者
for _ in range(2): # 模拟有两个线程
producer_thread = threading.Thread(target=producer.crawl)
consumer_thread = threading.Thread(target=consumer.process)
producer_thread.start()
consumer_thread.start()
# 加入结束标志
for _ in range(len(urls_to_crawl)):
content_queue.put(urls_to_crawl.pop())
# 等待所有任务完成
content_queue.join()
# 结束信号
producer.urls_queue.put(None) # 生产者结束
consumer.content_queue.put(None) # 消费者结束
```
在这个例子中,`Producer`和`Consumer`通过队列`content_queue`传递数据。当生产者爬取完所有URL后,会放入None作为结束信号,消费者接收到此信号后停止工作。
阅读全文
相关推荐
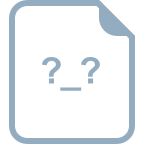
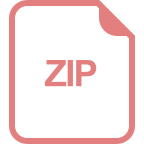
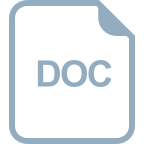
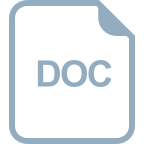
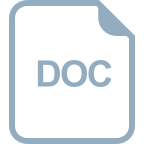
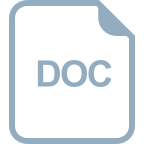
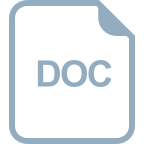
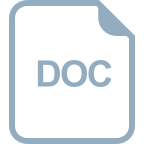
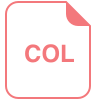
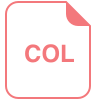
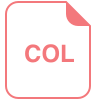
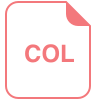
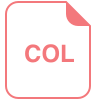
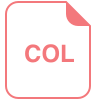
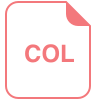
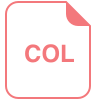
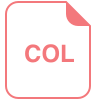
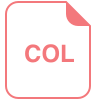