用C++完成下面的问题1.二分查找的非递归算法实现; 2.二分查找的递归算法实现; 3.基于有序序列的儿又判定树的构造; 4.尝试删除二叉排序树中的节点
时间: 2024-11-27 20:08:58 浏览: 46
1. **非递归二分查找**(C++实现):
```cpp
int binarySearchNonRecursive(int arr[], int target, int left, int right) {
if (left > right)
return -1;
int mid = left + (right - left) / 2;
if (arr[mid] == target)
return mid;
else if (arr[mid] < target)
return binarySearchNonRecursive(arr, target, mid+1, right);
else
return binarySearchNonRecursive(arr, target, left, mid-1);
}
```
2. **递归二分查找**(C++实现):
```cpp
int binarySearchRecursive(vector<int>& arr, int target, int start = 0, int end = arr.size() - 1) {
if (start > end)
return -1;
int mid = start + (end - start) / 2;
if (arr[mid] == target)
return mid;
else if (arr[mid] < target)
return binarySearchRecursive(arr, target, mid + 1, end);
else
return binarySearchRecursive(arr, target, start, mid - 1);
}
```
3. **构建二叉判定树**(C++实现,假设`Node`类表示节点):
```cpp
void buildSearchTree(Node* &root, const vector<int>& values) {
for (const auto& val : values) {
if (!root || root->value < val)
root = new Node{val, nullptr};
else {
Node* current = root;
while (current->right && current->right->value < val)
current = current->right;
current->right = new Node{val, current->right};
}
}
}
```
4. **删除二叉排序树节点**(C++实现):
```cpp
void removeNode(Node*& root, int key) {
if (!root)
return;
if (key < root->value)
root->left = removeNode(root->left, key);
else if (key > root->value)
root->right = removeNode(root->right, key);
else { // 找到要删除的节点
if (!root->left) {
swap(root, root->right);
delete root;
} else if (!root->right) {
swap(root, root->left);
delete root;
} else { // 有左右两个孩子
Node* temp = findMin(root->right); // 找右子树最小值
root->value = temp->value;
root->right = removeNode(root->right, temp->value);
}
}
}
```
阅读全文
相关推荐




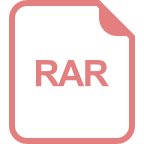









