单链表的前驱查找C++代码
时间: 2024-10-20 07:02:08 浏览: 39
单链表中的前驱节点查找通常是在给定一个目标节点后,找到该节点的直接前一个节点。这个操作可以通过遍历链表并记录每个节点来完成。以下是C++中简单的单链表前驱节点查找的一个示例:
```cpp
#include <iostream>
// 定义链表节点结构体
struct ListNode {
int val;
ListNode* next;
ListNode(int x) : val(x), next(NULL) {}
};
// 查找前驱节点的函数
ListNode* findPredecessor(ListNode* head, ListNode* target) {
if (head == nullptr || head->next == target)
return nullptr; // 如果头节点为空,或者头节点就是目标节点,则无前驱
ListNode* current = head->next; // 从头节点的下一个开始遍历
while (current != target) {
if (current->next == target) // 找到目标节点
return current; // 返回当前节点作为前驱
current = current->next;
}
return nullptr; // 目标节点不在链表中,返回nullptr
}
int main() {
// 创建链表示例
ListNode* list = new ListNode(1);
list->next = new ListNode(2);
list->next->next = new ListNode(3);
list->next->next->next = new ListNode(4);
ListNode* target = list->next->next; // 假设我们要找3的前驱
ListNode* predecessor = findPredecessor(list, target);
if (predecessor)
std::cout << "The predecessor of " << target->val << " is " << predecessor->val << std::endl;
else
std::cout << "Target node not found in the list" << std::endl;
delete list;
return 0;
}
```
阅读全文
相关推荐


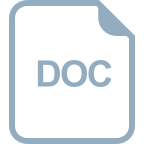















