python爬虫爬视频
时间: 2024-12-12 12:13:17 浏览: 15
Python爬虫可以用来爬取网页中的视频内容。以下是一个简单的步骤指南,帮助你使用Python爬取视频:
1. **安装必要的库**:
你需要安装一些常用的Python库,如`requests`用于发送HTTP请求,`BeautifulSoup`用于解析HTML,`youtube_dl`用于下载视频。
```bash
pip install requests beautifulsoup4 youtube_dl
```
2. **发送HTTP请求**:
使用`requests`库发送HTTP请求,获取网页内容。
```python
import requests
url = 'https://example.com/video-page'
response = requests.get(url)
if response.status_code == 200:
html_content = response.text
else:
print('Failed to retrieve the webpage')
```
3. **解析HTML内容**:
使用`BeautifulSoup`解析HTML内容,提取视频的URL。
```python
from bs4 import BeautifulSoup
soup = BeautifulSoup(html_content, 'html.parser')
video_tag = soup.find('video')
if video_tag:
video_url = video_tag.find('source')['src']
else:
video_url = soup.find('a', {'class': 'download-link'})['href']
```
4. **下载视频**:
使用`youtube_dl`库下载视频。
```python
import youtube_dl
ydl_opts = {
'outtmpl': 'downloads/%(title)s.%(ext)s',
}
with youtube_dl.YoutubeDL(ydl_opts) as ydl:
ydl.download([video_url])
```
5. **完整代码**:
将上述步骤整合在一起,形成一个完整的爬虫程序。
```python
import requests
from bs4 import BeautifulSoup
import youtube_dl
url = 'https://example.com/video-page'
response = requests.get(url)
if response.status_code == 200:
html_content = response.text
soup = BeautifulSoup(html_content, 'html.parser')
video_tag = soup.find('video')
if video_tag:
video_url = video_tag.find('source')['src']
else:
video_url = soup.find('a', {'class': 'download-link'})['href']
ydl_opts = {
'outtmpl': 'downloads/%(title)s.%(ext)s',
}
with youtube_dl.YoutubeDL(ydl_opts) as ydl:
ydl.download([video_url])
else:
print('Failed to retrieve the webpage')
```
阅读全文
相关推荐



















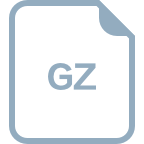