C# 35位 数字的 随机预测
时间: 2024-10-18 12:23:59 浏览: 18
在C#中,如果你想生成一个范围在0到999999999999999999(35位数字)之间的随机数,你可以利用`BigInteger`类,因为它能处理任意精度整数。这里是一个简单的示例:
```csharp
using System.Numerics;
public BigInteger GenerateRandom35DigitNumber()
{
Random random = new Random(); // 或者使用Guid.NewGuid()获取更高质量的随机数源
return new BigInteger(random.Next((long)Int64.MaxValue + 1)); // 考虑Int64的最大值再加上1避免溢出
}
// 使用生成的随机数
BigInteger randomNum = GenerateRandom35DigitNumber();
Console.WriteLine(randomNum.ToString()); // 输出随机35位数字
```
请注意,虽然理论上能生成35位的数字,但在实际应用中,由于计算机内存限制,可能无法显示完全35位的数值,特别是在控制台输出时。
相关问题
C# 35个 数字的 随机预测
在C#中,如果你想生成35个随机数字并进行预测,你可以使用`Random`类或者`System.Security.Cryptography.RandomNumberGenerator`类来实现。这里是一个基本的例子,使用`Random`类生成35个0到100之间的随机整数:
```csharp
using System;
using System.Collections.Generic;
class RandomPredictionExample
{
static void Main()
{
Random random = new Random();
List<int> randomNumbers = GenerateRandomNumbers(35, 0, 100);
Console.WriteLine("生成的35个随机数预测示例:");
foreach (int number in randomNumbers)
{
Console.Write(number + " ");
}
// 如果需要进行简单的预测分析,可以统计频率、平均值等,但这部分取决于具体的预测模型
// 在此不做详细展示。
}
static List<int> GenerateRandomNumbers(int count, int min, int max)
{
return Enumerable.Range(min, count).Select(_ => random.Next(max - min + 1) + min).ToList();
}
}
```
这个例子首先创建了一个随机数生成器,然后使用`Enumerable.Range`生成指定范围内的整数序列,接着使用`random.Next`将其转换为指定大小的随机数列表。
C# 35个数字 中 的5个数字出现历史记录 预测 下一次的出现概率 完整代码
在C#中,预测特定数字序列下一次出现的概率通常涉及到统计分析和随机数生成。由于这是一个基于概率的预测,并非机器学习模型或复杂的算法,这里我们假设有一个简单的随机数据集,我们可以使用`System.Random`类来进行模拟。
首先,你需要存储历史记录的数字以及它们出现的次数。这可以通过字典(Dictionary<TKey, TValue>)来实现,键是数字,值是出现次数。然后你可以计算每个数字出现的频率,最后基于这个频率估算下次出现的概率。
下面是一个简单的示例代码:
```csharp
using System;
using System.Collections.Generic;
class Program
{
static Dictionary<int, int> historicalRecords = new Dictionary<int, int>();
static Random random = new Random();
static void Main()
{
// 假设这里有5个数字的历史记录
for (int i = 0; i < 35; i++)
{
int nextNumber = GenerateRandomNumber();
if (!historicalRecords.ContainsKey(nextNumber))
historicalRecords[nextNumber] = 1;
else
historicalRecords[nextNumber]++;
}
foreach (KeyValuePair<int, int> record in historicalRecords.OrderByDescending(pair => pair.Value))
{
double probability = (double)record.Value / 35; // 假定35次抽样
Console.WriteLine($"数字 {record.Key} 出现了 {record.Value} 次,概率大约为 {probability * 100}%");
}
// 预测下一次出现的概率
int predictedNumber = GenerateRandomNumberWithProbability(historicalRecords);
Console.WriteLine($"根据历史,下一次最有可能出现的数字是 {predictedNumber}");
}
static int GenerateRandomNumber()
{
return random.Next(0, 36); // 假设0-35之间的数字
}
static int GenerateRandomNumberWithProbability(Dictionary<int, int> records)
{
List<KeyValuePair<int, int>> sortedList = records.ToList();
sortedList.Sort((a, b) => b.Value.CompareTo(a.Value)); // 按照概率降序排序
double totalProb = 0;
foreach (var item in sortedList)
{
totalProb += item.Value / 35;
if (random.NextDouble() <= totalProb)
return item.Key;
}
return sortedList[sortedList.Count - 1].Key; // 如果所有概率加起来超过1,返回最大概率的那个
}
}
```
注意,此代码只是一个简化的例子,实际应用中可能需要处理更复杂的数据源、更精确的频率估计,或者使用更专业的统计模型。
阅读全文
相关推荐
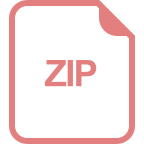
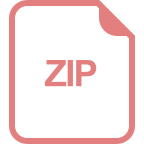
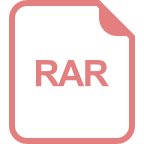
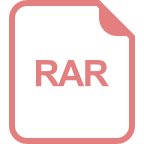
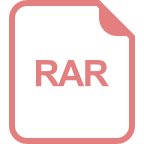
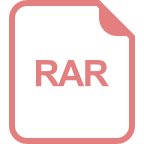
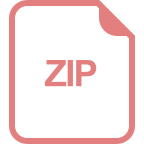
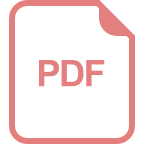

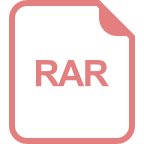
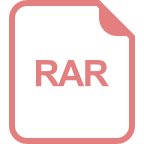
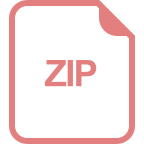
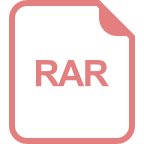
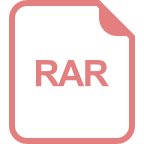
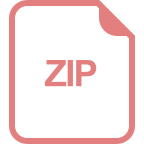