用dev-c++编写程序,用函数实现九九乘法口诀表并输出,九列九行的效果
时间: 2024-11-09 21:30:18 浏览: 32
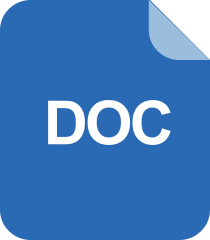
使用Dev-C++建立新项目的操作步骤

在Dev-C++中编写一个九九乘法口诀表的函数,可以按照以下步骤操作:
首先,创建一个新的C++源文件,例如`multiplication_table.cpp`。然后,在这个文件里,你可以定义一个函数`print_multiplication_table()`,该函数会生成并打印出九九乘法表。
```cpp
#include <iostream>
using namespace std;
// 定义函数,用于打印乘法口诀表
void print_multiplication_table() {
for (int i = 1; i <= 9; ++i) { // 遍历1到9
for (int j = 1; j <= i; ++j) { // 内层循环从1到当前行数
cout << j << " * " << i << " = " << i*j << "\t"; // 输出乘法表达式和结果
}
cout << endl; // 每行结束后换行
}
}
int main() {
print_multiplication_table();
return 0;
}
```
当你运行这个程序,它会在控制台输出九九乘法口诀表,每行代表乘积的一列,共九列九行。
阅读全文
相关推荐
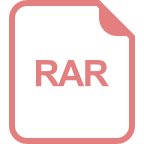



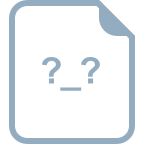








