js正则校验和指定字符串完全一致
时间: 2023-09-02 07:15:09 浏览: 138
要校验一个字符串是否与指定字符串完全一致,你可以使用精确匹配的正则表达式。以下是一个示例:
```javascript
function validateString(input, specifiedString) {
// 使用正则表达式匹配规则
var regex = new RegExp(`^${specifiedString}$`);
return regex.test(input);
}
// 测试示例
console.log(validateString("Test", "Test")); // true
console.log(validateString("Hello World", "World")); // false
console.log(validateString("123", "Test")); // false
console.log(validateString("@Test", "Test")); // false
```
在上面的示例中,`validateString` 函数接收两个参数:`input` 是输入字符串,`specifiedString` 是指定的字符串。函数使用了精确匹配的正则表达式 `new RegExp(`^${specifiedString}$`)` 来匹配输入字符串。这个正则表达式的含义是:输入字符串必须与指定的字符串完全一致。如果输入字符串与指定字符串完全一致,则返回 `true`,否则返回 `false`。
你可以根据需要修改指定的字符串或调整校验的规则。
相关问题
语言javascript,将一串字符串正则校验获取该字符串中符合正则校验规则的代码怎么写
你可以使用 JavaScript 中的正则表达式对象 `RegExp`,以及字符串对象的 `match` 方法来实现字符串正则校验并获取符合规则的代码。
以下是一个示例代码,演示如何获取一个字符串中所有符合正则表达式 `/function\s+\w+\s*\(/g` 的函数定义代码:
```javascript
const str = 'function foo() { console.log("hello world"); } function bar() { alert("hello!"); }';
const regex = /function\s+\w+\s*\(/g;
const matches = str.match(regex);
if (matches !== null) {
console.log(matches);
} else {
console.log("No matches found.");
}
```
这段代码会输出两个函数定义代码:`function foo() { console.log("hello world"); }` 和 `function bar() { alert("hello!"); }`。你可以根据你的具体需求修改正则表达式和代码逻辑。
js正则校验去除特殊字符
你好!针对你的问题,可以使用以下正则表达式来去除字符串中的特殊字符:
```javascript
str.replace(/[^\w\u4e00-\u9fa5]/gi, '')
```
其中,`\w` 匹配所有数字、字母和下划线,`\u4e00-\u9fa5` 匹配所有中文字符,`[]` 代表字符集合,`^` 代表取反,`/gi` 表示全局匹配,并且不区分大小写。
例如,以下代码可以使用该正则表达式来去除一个字符串中的特殊字符:
```javascript
const str = 'hello#world!你好,世界!123';
const newStr = str.replace(/[^\w\u4e00-\u9fa5]/gi, ''); // 去除特殊字符
console.log(newStr); // helloworld你好世界123
```
这样,就可以将特殊字符去除,得到一个只包含数字、字母和中文字符的新字符串。
阅读全文
相关推荐

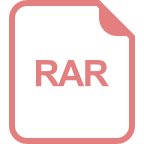












