使用c语言实现模拟凯撒密码
时间: 2024-10-21 20:07:34 浏览: 31
凯撒密码是一种简单的加密技术,通过将明文中的每个字母按照固定位移(密钥值)进行替换来达到加密的目的。以下是使用 C 语言实现凯撒密码的一个简单示例:
```c
#include <stdio.h>
#include <ctype.h>
// 加密函数,字母移动指定位数
char caesar_encrypt(char c, int key) {
if (isalpha(c)) { // 检查字符是否为字母
if (islower(c))
return ((c - 'a' + key) % 26) + 'a'; // 对小写字母进行加密
else
return ((c - 'A' + key) % 26) + 'A'; // 对大写字母进行加密
}
// 非字母字符保持不变
return c;
}
int main() {
char plaintext[100], ciphertext[100];
int key, i;
printf("请输入明文:\n");
fgets(plaintext, sizeof(plaintext), stdin);
plaintext[strlen(plaintext) - 1] = '\0'; // 去除换行符
printf("请输入密钥值:\n");
scanf("%d", &key);
for (i = 0; plaintext[i]; ++i)
ciphertext[i] = caesar_encrypt(plaintext[i], key);
ciphertext[strlen(ciphertext)] = '\0';
printf("加密后的密文为: %s\n", ciphertext);
return 0;
}
```
在这个程序中,`caesar_encrypt` 函数接收一个字符 `c` 和一个密钥 `key`,然后根据字母的大小写分别进行加密。用户会被提示输入明文和密钥,程序会处理每一字符并输出加密后的结果。
阅读全文
相关推荐
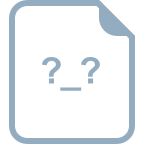
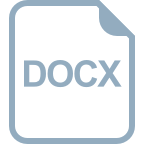
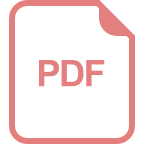


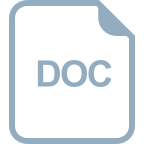
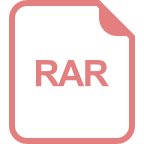
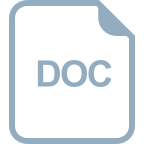
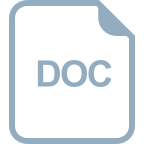
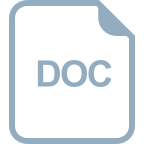
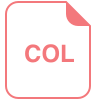
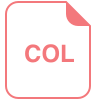


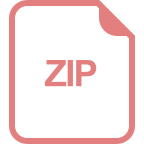
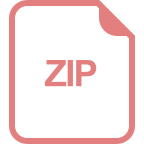
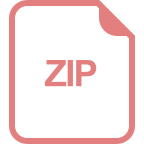
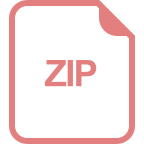