C++高精度加法函数
时间: 2024-10-03 07:01:55 浏览: 35
在C++中,实现高精度加法通常涉及到自定义数据结构来存储大整数,并编写函数来进行逐位相加操作。这通常不是标准库的一部分,因为C++的标准库(如`<iostream>`或`<iomanip>`) 提供的是有限的整数类型,对于非常大的数值处理可能存在溢出的问题。
下面是一个简单的示例,展示了如何创建一个基本的高精度加法函数:
```cpp
#include <vector>
class BigInt {
private:
std::vector<unsigned char> digits;
public:
// 构造函数,添加0作为初始值
BigInt() : digits(1, 0) {}
// 加法运算,手动实现逐位相加
BigInt operator+(const BigInt& other) const {
BigInt result = *this;
if (result.digits.size() < other.digits.size()) {
result.digits.insert(result.digits.begin(), other.digits.size() - result.digits.size(), 0);
} else if (other.digits.size() < result.digits.size()) {
other.digits.insert(other.digits.begin(), result.digits.size() - other.digits.size(), 0);
}
unsigned carry = 0;
for (size_t i = 0; i < result.digits.size(); ++i) {
result.digits[i] += other.digits[i] + carry;
carry = result.digits[i] > 9;
if (carry) {
result.digits[i] -= 10;
}
}
// 如果还有进位,将它添加到第一个位置
while (carry) {
result.digits.insert(result.digits.begin(), carry);
carry = 0;
}
return result;
}
};
// 示例用法
int main() {
BigInt a("123456789");
BigInt b("987654321");
BigInt sum = a + b;
// 输出结果...
}
```
阅读全文
相关推荐
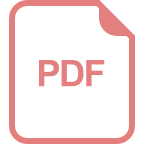
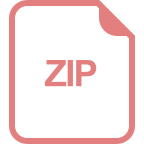
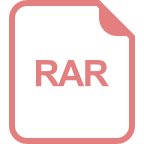





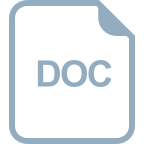
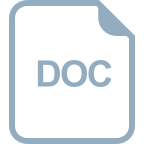






