用c#实现读取一个txt文件并将里面的id写入到list中
时间: 2024-11-22 15:41:02 浏览: 27
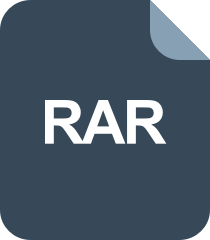
C# 读取一个文件夹中多个txt,去重正则化后重新写入,要求每个不能超过二十万行
在C#中,你可以使用`StreamReader`类来逐行读取.txt文件的内容,并将每一行的ID添加到`List<int>`中。以下是一个简单的示例:
```csharp
using System;
using System.Collections.Generic;
using System.IO;
public class Program
{
public static void Main()
{
string filePath = "path_to_your_file.txt"; // 替换为实际的文件路径
List<int> ids = new List<int>();
try
{
using (StreamReader reader = new StreamReader(filePath))
{
string line;
while ((line = reader.ReadLine()) != null)
{
int id;
if (int.TryParse(line, out id)) // 检查是否能转换为整数
{
ids.Add(id);
}
else
{
Console.WriteLine($"无法解析ID:{line}");
}
}
}
// 输出或进一步处理ids列表
Console.WriteLine("IDs已读取并添加到列表中");
}
catch (Exception ex)
{
Console.WriteLine($"错误发生:{ex.Message}");
}
}
}
```
在这个示例中,我们首先创建一个`StreamReader`来打开文件,然后通过`ReadLine()`方法逐行读取。如果每行可以成功转换为整数,我们就将其添加到`List<int>`中。如果无法转换,我们将忽略这一行并打印一条错误消息。
阅读全文
相关推荐
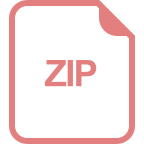
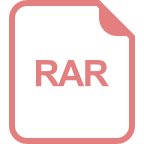
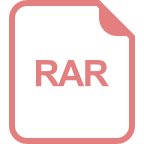
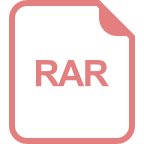
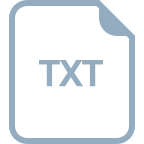
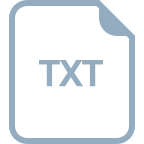
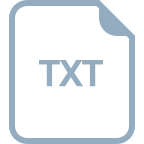
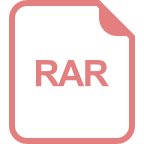
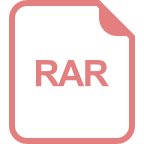
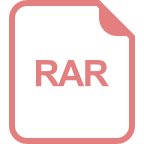
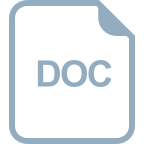
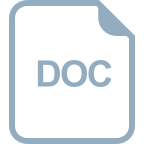
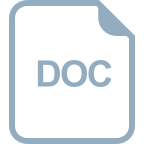




