Exception in thread Thread-1: Traceback (most recent call last): File "D:\python\python3.7\lib\threading.py", line 926, in _bootstrap_inner self.run() File "D:\python\python3.7\lib\threading.py", line 870, in run self._target(*self._args, **self._kwargs) File "D:\python\python3.7\lib\tkinter\__init__.py", line 1489, in cget return self.tk.call(self._w, 'cget', '-' + key) TypeError: can only concatenate str (not "int") to str
时间: 2023-09-13 16:11:01 浏览: 445
这个错误是由于在使用 `tkinter` 中的 `Label` 组件时,传递给 `image` 参数的值应该是一个字符串,而不是一个整数。
在更新图像的代码中,你可能会遇到以下问题:
```python
photo = queue.get()
canvas.config(image=photo)
```
`config()` 方法的 `image` 参数需要传递一个字符串类型的值,而不是一个 `PhotoImage` 对象。为了解决这个问题,你可以使用实例变量来保存 `PhotoImage` 对象,然后在 `config()` 方法中使用该变量。
以下是修正后的代码示例:
```python
import tkinter as tk
import cv2
from PIL import Image, ImageTk
import threading
from queue import Queue
def start_camera(queue):
cap = cv2.VideoCapture(0)
while True:
ret, frame = cap.read()
if not ret:
break
frame = cv2.cvtColor(frame, cv2.COLOR_BGR2RGB)
image = Image.fromarray(frame)
image = image.resize((640, 480))
photo = ImageTk.PhotoImage(image)
queue.put(photo)
if cv2.waitKey(1) & 0xFF == ord('q'):
break
cap.release()
def update_frame(queue):
while True:
photo = queue.get()
canvas.image = photo # 保存 PhotoImage 对象到实例变量
canvas.config(image=canvas.image) # 使用实例变量作为参数
def start_thread():
queue = Queue()
camera_thread = threading.Thread(target=start_camera, args=(queue,))
frame_thread = threading.Thread(target=update_frame, args=(queue,))
camera_thread.start()
frame_thread.start()
root = tk.Tk()
canvas = tk.Label(root)
canvas.pack()
button = tk.Button(root, text="启动摄像头", command=start_thread)
button.pack()
root.mainloop()
```
在修正后的代码中,我们将 `PhotoImage` 对象保存到 `canvas.image` 实例变量中,在更新图像时使用该变量作为参数传递给 `config()` 方法,避免了类型错误。
阅读全文
相关推荐
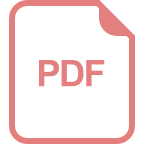
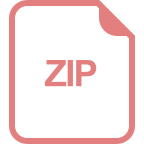
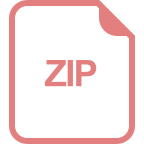
















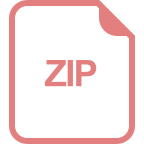