在js中实现数据左右链接
时间: 2024-10-23 13:15:03 浏览: 13
在JavaScript中实现数据的左右链接通常涉及到数组的操作和链表的概念。如果你想要创建一个简单的双向链表结构,可以定义两个节点属性:`prev`(前一个节点引用)和`next`(下一个节点引用),并提供添加、删除节点等基本操作。下面是一个简单的示例:
```javascript
// 定义链表节点
class Node {
constructor(data) {
this.data = data;
this.prev = null; // 指向前一个节点
this.next = null; // 指向后一个节点
}
}
// 双向链表类
class DoublyLinkedList {
constructor() {
this.head = null;
this.tail = null;
}
// 添加节点到链表尾部
append(data) {
const newNode = new Node(data);
if (!this.head) { // 如果链表为空
this.head = newNode;
this.tail = newNode;
} else {
newNode.prev = this.tail;
this.tail.next = newNode;
this.tail = newNode;
}
}
// 在头部添加节点
prepend(data) {
const newNode = new Node(data);
newNode.next = this.head;
if (this.head) {
this.head.prev = newNode;
}
this.head = newNode;
}
// 删除指定节点(这里仅删除头节点作为简化示例)
removeFirst() {
if (this.head) {
const nodeToRemove = this.head;
this.head = nodeToRemove.next;
if (this.head) {
this.head.prev = null;
} else {
this.tail = null;
}
nodeToRemove.next = null; // 清理旧节点的引用
}
}
}
// 使用示例
const list = new DoublyLinkedList();
list.append(1);
list.prepend(0);
console.log(list); // 输出:[0 -> 1]
// 删除第一个元素
list.removeFirst();
console.log(list); // 输出:[1]
```
阅读全文
相关推荐
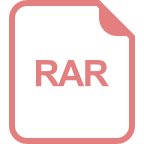
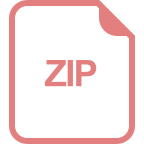
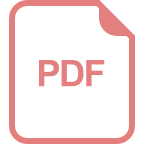
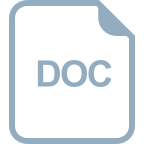
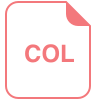
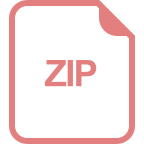
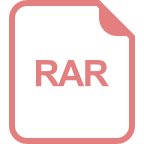
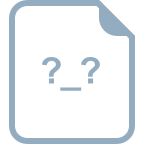
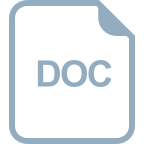
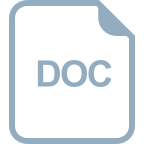
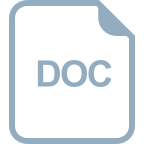
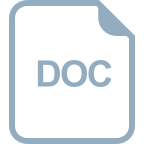
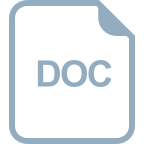
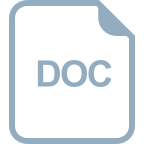
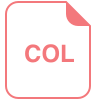
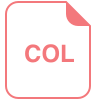
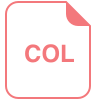
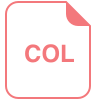
