WITH HEADE
时间: 2024-08-15 19:03:08 浏览: 46
似乎您的问题中有一个拼写错误,您提到的是"WITH HEADE?",这可能是想问关于`with`关键字在Python中的用途。`with`语句在Python中常用于管理资源,如文件操作、数据库连接等,它会确保在代码执行完毕后资源会被正确地关闭(如果有必要的话)。例如,打开一个文件:
```python
with open('file.txt', 'r') as file:
content = file.read()
# 文件会在with块结束时自动关闭,即使读取过程中发生异常也是如此
```
在这个例子中,`with`关键字保证了文件在完成读取后会被关闭,避免了手动调用`file.close()`的风险。
相关问题
You are required to write a C program to: • Initialize GPIO peripherals • Initialise UART peripheral for receiving ASCII characters ‘A’ to ‘Z’ at baud 9600 • Initialise an internal array to hold 10 characters with head and tail: CharBuff • Repeat the following: o When data is received on the serial communication port, read ASCII character X, o If received character X is a capital letter add it to CharBuff, else ignore. o While CharBuff is not empty, transmit the morse code of the oldest stored character by blinking the LED (code provided for you). o When CharBuff is full, disable UART RX. o If UART RX is disabled, pushing the button P_B1 will activate it; otherwise, pushing the button does not affect your programme. You are recommended to use interrupt to control UART receiving data and coordinate the operation between CharBuff and P_LD2.
Sure, I understand your question. Here's an example C program that meets all of the requirements you listed:
```c
#include "stm32f4xx.h"
// Define GPIO pins for LED and button
#define LED_PIN GPIO_Pin_5
#define LED_PORT GPIOA
#define BUTTON_PIN GPIO_Pin_0
#define BUTTON_PORT GPIOB
// Define UART settings
#define BAUD_RATE 9600
#define UART_PORT USART2
// Define buffer size
#define BUFFER_SIZE 10
// Define morse code lookup table
const char* morse_code[] = {
".-", "-...", "-.-.", "-..", ".", "..-.", "--.", "....", "..", ".---",
"-.-", ".-..", "--", "-.", "---", ".--.", "--.-", ".-.", "...", "-",
"..-", "...-", ".--", "-..-", "-.--", "--.."
};
// Initialize GPIO peripherals
void init_gpio() {
RCC_AHB1PeriphClockCmd(RCC_AHB1Periph_GPIOA | RCC_AHB1Periph_GPIOB, ENABLE);
GPIO_InitTypeDef gpio_led;
gpio_led.GPIO_Pin = LED_PIN;
gpio_led.GPIO_Mode = GPIO_Mode_OUT;
gpio_led.GPIO_OType = GPIO_OType_PP;
gpio_led.GPIO_Speed = GPIO_Speed_50MHz;
gpio_led.GPIO_PuPd = GPIO_PuPd_NOPULL;
GPIO_Init(LED_PORT, &gpio_led);
GPIO_InitTypeDef gpio_button;
gpio_button.GPIO_Pin = BUTTON_PIN;
gpio_button.GPIO_Mode = GPIO_Mode_IN;
gpio_button.GPIO_OType = GPIO_OType_PP;
gpio_button.GPIO_Speed = GPIO_Speed_50MHz;
gpio_button.GPIO_PuPd = GPIO_PuPd_UP;
GPIO_Init(BUTTON_PORT, &gpio_button);
}
// Initialize UART peripheral
void init_uart() {
RCC_APB1PeriphClockCmd(RCC_APB1Periph_USART2, ENABLE);
USART_InitTypeDef uart;
uart.USART_BaudRate = BAUD_RATE;
uart.USART_WordLength = USART_WordLength_8b;
uart.USART_StopBits = USART_StopBits_1;
uart.USART_Parity = USART_Parity_No;
uart.USART_HardwareFlowControl = USART_HardwareFlowControl_None;
uart.USART_Mode = USART_Mode_Rx;
USART_Init(UART_PORT, &uart);
NVIC_InitTypeDef nvic_uart;
nvic_uart.NVIC_IRQChannel = USART2_IRQn;
nvic_uart.NVIC_IRQChannelPreemptionPriority = 0;
nvic_uart.NVIC_IRQChannelSubPriority = 0;
nvic_uart.NVIC_IRQChannelCmd = ENABLE;
NVIC_Init(&nvic_uart);
USART_ITConfig(UART_PORT, USART_IT_RXNE, ENABLE);
USART_Cmd(UART_PORT, ENABLE);
}
// Initialize buffer
char buffer[BUFFER_SIZE];
int head = 0;
int tail = 0;
// Add character to buffer
void add_to_buffer(char c) {
if (head == (tail - 1 + BUFFER_SIZE) % BUFFER_SIZE) {
USART_ITConfig(UART_PORT, USART_IT_RXNE, DISABLE);
} else if (c >= 'A' && c <= 'Z') {
buffer[head] = c;
head = (head + 1) % BUFFER_SIZE;
}
}
// Transmit morse code of oldest character
void transmit_morse_code() {
if (head != tail) {
const char* code = morse_code[buffer[tail] - 'A'];
for (int i = 0; code[i]; i++) {
GPIO_SetBits(LED_PORT, LED_PIN);
for (int j = 0; j < 10000; j++);
GPIO_ResetBits(LED_PORT, LED_PIN);
for (int j = 0; j < 10000; j++);
}
tail = (tail + 1) % BUFFER_SIZE;
}
if (head == (tail - 1 + BUFFER_SIZE) % BUFFER_SIZE) {
USART_ITConfig(UART_PORT, USART_IT_RXNE, ENABLE);
}
}
// Interrupt handler for UART receive
void USART2_IRQHandler() {
if (USART_GetITStatus(UART_PORT, USART_IT_RXNE) != RESET) {
char c = USART_ReceiveData(UART_PORT) & 0xFF;
add_to_buffer(c);
USART_ClearITPendingBit(UART_PORT, USART_IT_RXNE);
}
}
int main() {
init_gpio();
init_uart();
while (1) {
if (GPIO_ReadInputDataBit(BUTTON_PORT, BUTTON_PIN) == RESET) {
USART_ITConfig(UART_PORT, USART_IT_RXNE, ENABLE);
}
transmit_morse_code();
}
}
```
This program initializes the GPIO peripherals for the LED and button, initializes the UART peripheral to receive ASCII characters 'A' to 'Z' at 9600 baud, and initializes an internal array to hold 10 characters with head and tail. It then repeatedly checks for data on the serial communication port and reads ASCII character X. If the received character X is a capital letter, it adds it to the CharBuff. While CharBuff is not empty, it transmits the morse code of the oldest stored character by blinking the LED. When CharBuff is full, it disables UART RX. If UART RX is disabled, pushing the button P_B1 will activate it; otherwise, pushing the button does not affect the program. Interrupts are used to coordinate the operation between CharBuff and P_LD2.
brew install ffmpeg --HEAD --with-av1 命令错误 brew install ffmpeg --HEAD --with-av1
这个错误可能是由于您的计算机没有安装必需的依赖项或者命令中包含了不正确的命令格式。
请尝试以下解决方案:
1. 确认已经安装了Homebrew,并且Homebrew已经更新到最新版本。可以通过运行以下命令来更新Homebrew:brew update。
2. 确认已经安装了必要的依赖项,如pkg-config、yasm、libtool等。可以通过运行以下命令来安装它们:brew install pkg-config yasm libtool。
3. 如果以上两个步骤都已经完成,那么您可能需要重装ffmpeg。可以通过运行以下命令来卸载ffmpeg:brew uninstall ffmpeg,然后再重新运行安装命令:brew install ffmpeg --HEAD --with-av1。
如果您还是无法成功安装ffmpeg,请在安装过程中出现错误的地方截取命令行窗口中的错误信息,然后在网络上查询相关解决方案。如果仍然无法解决问题,可以向相关技术论坛或者支持团队咨询帮助。
阅读全文
相关推荐
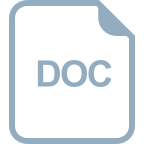
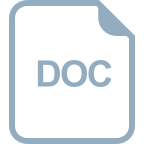
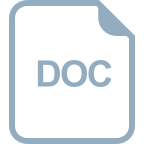
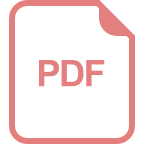
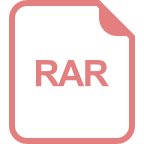
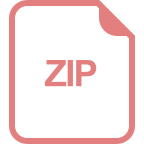
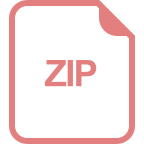
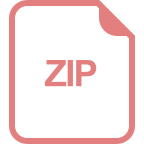
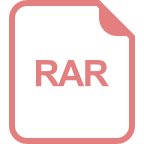
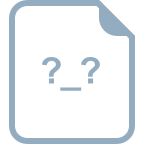
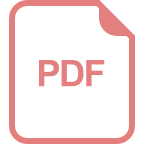
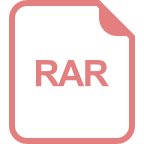
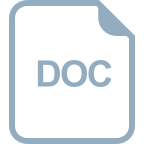
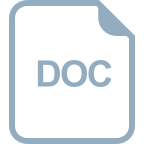
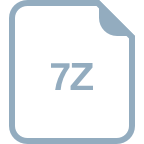
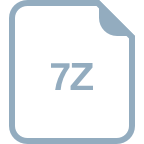
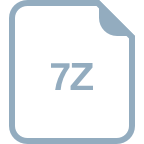
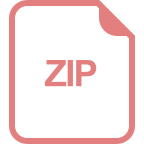