opencv数字识别算法代码
时间: 2024-03-07 14:45:55 浏览: 17
基于OpenCV的数字识别算法代码如下所示[^1]:
```cpp
#include <iostream>
#include <fstream>
#include <sstream>
#include <vector>
#include <opencv2/opencv.hpp>
#include <opencv2/ml.hpp>
using namespace std;
using namespace cv;
using namespace cv::ml;
// 读取训练数据
void readData(const string& filename, Mat& data, Mat& labels) {
ifstream file(filename);
if (!file.is_open()) {
cerr << "无法打开文件: " << filename << endl;
exit(-1);
}
vector<vector<float>> values;
string line;
while (getline(file, line)) {
stringstream ss(line);
vector<float> row;
float value;
while (ss >> value) {
row.push_back(value);
}
values.push_back(row);
}
data = Mat(values.size(), values[0].size() - 1, CV_32F);
labels = Mat(values.size(), 1, CV_32S);
for (int i = 0; i < values.size(); i++) {
for (int j = 0; j < values[i].size() - 1; j++) {
data.at<float>(i, j) = values[i][j];
}
labels.at<int>(i, 0) = values[i][values[i].size() - 1];
}
}
int main() {
// 读取训练数据
Mat trainData, trainLabels;
readData("train.txt", trainData, trainLabels);
// 创建SVM分类器
Ptr<SVM> svm = SVM::create();
svm->setType(SVM::C_SVC);
svm->setKernel(SVM::LINEAR);
svm->setTermCriteria(TermCriteria(TermCriteria::MAX_ITER, 100, 1e-6));
// 训练SVM分类器
svm->train(trainData, ROW_SAMPLE, trainLabels);
// 保存训练好的模型
svm->save("svm_model.xml");
// 加载测试数据
Mat testData, testLabels;
readData("test.txt", testData, testLabels);
// 预测测试数据
Mat predictions;
svm->predict(testData, predictions);
// 计算准确率
int correct = 0;
for (int i = 0; i < predictions.rows; i++) {
if (predictions.at<float>(i, 0) == testLabels.at<int>(i, 0)) {
correct++;
}
}
float accuracy = (float)correct / predictions.rows * 100;
cout << "准确率: " << accuracy << "%" << endl;
return 0;
}
```
请注意,这只是一个示例代码,实际使用时需要根据具体情况进行修改和调整。
相关推荐
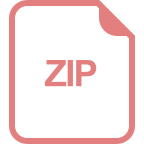
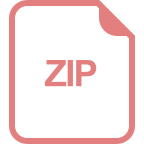
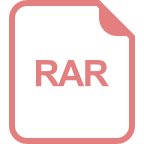














