vue使用openlayers绘制线用图片展示
时间: 2024-08-20 22:01:14 浏览: 52
在Vue项目中使用OpenLayers绘制线条,并且用图片来展示,可以通过以下步骤实现:
1. 安装OpenLayers:首先确保你已经安装了Node.js,然后在项目中安装OpenLayers库,可以使用npm或yarn命令行工具来安装。
```
npm install ol
```
或者
```
yarn add ol
```
2. 创建Vue组件:在你的Vue项目中创建一个新的组件,并导入OpenLayers相关的模块。
3. 绘制线条:在Vue组件的`<template>`部分,你可以使用OpenLayers提供的`<ol-map>`和`<ol-view>`等组件来创建地图。在`<ol-map>`内部,你可以使用`<ol-tile-layer>`来加载地图瓦片,`<ol-vector-layer>`来添加矢量图层,然后在矢量图层上绘制线条。
4. 使用图片标记线条:要在绘制的线条上使用图片作为标记,可以使用`<ol-style>`和`<ol-image>`来自定义矢量图层的样式。`<ol-image>`元素允许你指定一个图片的URL作为标记。
下面是一个简化的示例代码,展示了如何在Vue组件中使用OpenLayers绘制线条并用图片标记:
```html
<template>
<div id="map" style="width: 100%; height: 400px;"></div>
</template>
<script>
import 'ol/ol.css';
import Map from 'ol/Map';
import View from 'ol/View';
import TileLayer from 'ol/layer/Tile';
import OSM from 'ol/source/OSM';
import VectorLayer from 'ol/layer/Vector';
import VectorSource from 'ol/source/Vector';
import Style from 'ol/style/Style';
import Image from 'ol/style/Image';
import LineString from 'ol/geom/LineString';
import Feature from 'ol/Feature';
export default {
name: 'OpenLayersMap',
data() {
return {
map: null,
vectorLayer: null,
vectorSource: null,
};
},
mounted() {
this.vectorLayer = new VectorLayer({
source: this.vectorSource,
});
this.map = new Map({
target: 'map',
layers: [
new TileLayer({
source: new OSM(),
}),
this.vectorLayer,
],
view: new View({
center: [0, 0],
zoom: 2,
}),
});
this.vectorSource = new VectorSource({
features: [
new Feature({
type: 'LineString',
coordinates: [
[0, 0],
[100, 100],
],
}),
],
});
this.vectorLayer.setStyle(
new Style({
stroke: new Stroke({
color: '#ff0000',
width: 3,
}),
image: new Image({
src: 'path/to/your/image.png',
size: [32, 32],
anchor: [0.5, 0.5],
}),
})
);
},
};
</script>
<style scoped>
#map {
width: 100%;
height: 100%;
}
</style>
```
请根据你的项目实际路径替换`path/to/your/image.png`为你的图片路径。此代码仅为示例,具体细节可能需要根据实际需求调整。
阅读全文
相关推荐
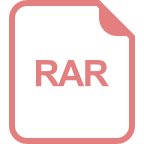
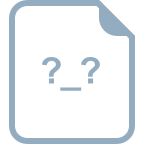
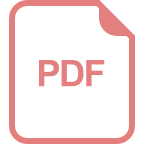
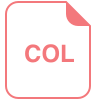
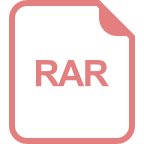
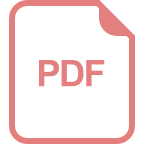
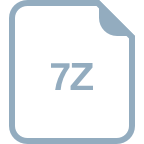
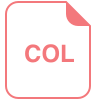
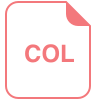
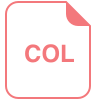
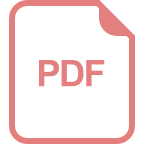
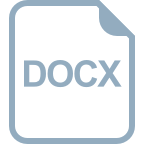
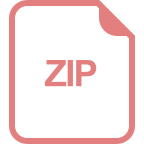
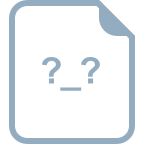
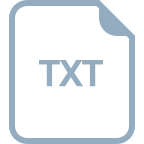
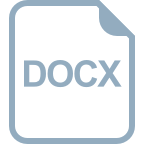
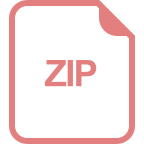