不要用RandomForestClassifier类,用底层代码搭建一个随机森林的算法,并且加入特征的随机选取
时间: 2024-04-11 09:27:17 浏览: 70
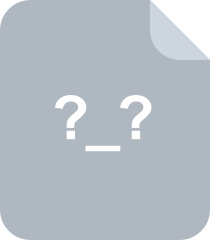
随机森林代码,用于特征筛选
明白了,你想要使用底层代码自己搭建一个随机森林算法,并且加入特征的随机选取。下面是一个示例代码,演示了如何实现:
```python
import numpy as np
class DecisionTree:
def __init__(self):
self.feature_indices = None
self.tree = None
def fit(self, X, y):
# 在这里编写决策树的训练代码
# ...
def predict(self, X):
# 在这里编写决策树的预测代码
# ...
return y_pred
class RandomForest:
def __init__(self, n_estimators=100, max_features=None, random_state=None):
self.n_estimators = n_estimators
self.max_features = max_features
self.random_state = random_state
self.trees = []
def fit(self, X, y):
np.random.seed(self.random_state)
for _ in range(self.n_estimators):
# 随机选择特征子集
if self.max_features is None:
num_features = X.shape[1]
else:
num_features = min(self.max_features, X.shape[1])
feature_indices = np.random.choice(X.shape[1], size=num_features, replace=False)
# 使用特征子集构建决策树
tree = DecisionTree()
tree.feature_indices = feature_indices
tree.fit(X[:, feature_indices], y)
# 将训练好的决策树添加到随机森林中
self.trees.append(tree)
def predict(self, X):
predictions = np.zeros((X.shape[0], len(self.trees)))
for i, tree in enumerate(self.trees):
feature_indices = tree.feature_indices
predictions[:, i] = tree.predict(X[:, feature_indices])
y_pred = np.mean(predictions, axis=1)
return y_pred
# 准备训练数据和目标变量
X_train = ...
y_train = ...
# 创建随机森林分类器
rf_classifier = RandomForest(n_estimators=100, max_features=3, random_state=42)
# 训练模型
rf_classifier.fit(X_train, y_train)
# 使用模型进行预测
X_test = ...
y_pred = rf_classifier.predict(X_test)
```
在上面的示例中,我们首先定义了一个`DecisionTree`类来实现决策树的训练和预测功能。在随机森林的训练过程中,我们在每个决策树的训练中随机选择一部分特征进行训练。这里使用了`np.random.choice`函数来进行特征的随机选择。然后,定义了一个`RandomForest`类来实现随机森林的训练和预测功能。在训练过程中,我们使用特征子集构建决策树,并将训练好的决策树添加到随机森林中。最后,使用随机森林对测试数据进行预测。
希望对你有所帮助!如果有其他问题,请随时提问。
阅读全文
相关推荐
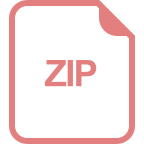
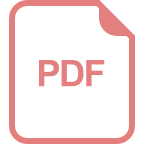
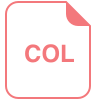
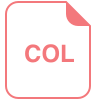
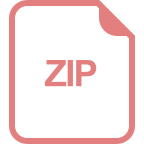
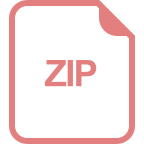
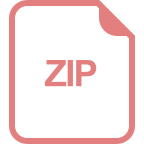
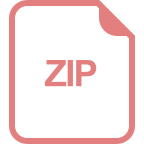
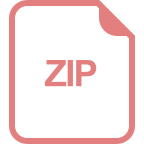
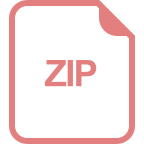
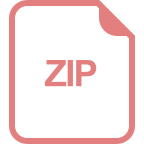
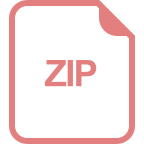
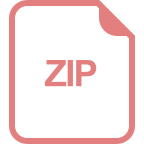
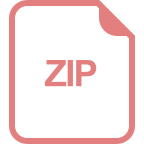
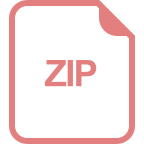
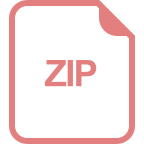
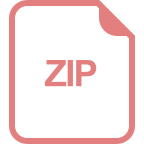
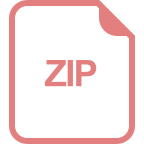